video swin transformer 代码
时间: 2023-07-01 22:26:55 浏览: 176
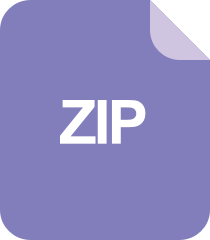
transformer代码

以下是使用3D卷积实现的Video Swin Transformer的代码示例,供您参考:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class VideoSwinTransformerBlock(nn.Module):
def __init__(self, in_channels, out_channels, num_heads, window_size, drop_rate=0.0):
super().__init__()
self.norm1 = nn.LayerNorm(in_channels)
self.attn = nn.MultiheadAttention(in_channels, num_heads)
self.norm2 = nn.LayerNorm(in_channels)
self.mlp = nn.Sequential(
nn.Linear(in_channels, out_channels),
nn.GELU(),
nn.Dropout(drop_rate),
nn.Linear(out_channels, in_channels),
nn.Dropout(drop_rate)
)
self.window_size = window_size
def forward(self, x):
# reshape input for 3D convolution
b, t, c, h, w = x.size()
x = x.view(b*t, c, h, w)
# add padding to input for overlapping window
p = self.window_size // 2
x = F.pad(x, (p, p, p, p), mode='reflect')
# apply 3D convolution with overlapping window
x = self.conv(x)
x = x.unfold(2, self.window_size, 1).unfold(3, self.window_size, 1)
x = x.permute(0, 2, 3, 4, 1, 5, 6).contiguous()
x = x.view(b*t*h*w, -1, c)
# apply transformer block
x = self.norm1(x)
attn_output, _ = self.attn(x, x, x)
x = x + attn_output
x = self.norm2(x)
x = self.mlp(x)
x = x.view(b*t, h, w, -1, c).permute(0, 3, 4, 1, 2).contiguous()
return x
class VideoSwinTransformer(nn.Module):
def __init__(self, in_channels, out_channels, num_heads, window_sizes, num_layers, drop_rate=0.0):
super().__init__()
self.conv = nn.Sequential(
nn.Conv3d(in_channels, out_channels, kernel_size=(1, 3, 3), stride=(1, 2, 2), padding=(0, 1, 1)),
nn.BatchNorm3d(out_channels),
nn.ReLU(inplace=True)
)
self.blocks = nn.ModuleList([
VideoSwinTransformerBlock(out_channels, out_channels, num_heads, window_size, drop_rate=drop_rate)
for window_size in window_sizes
])
self.norm = nn.LayerNorm(out_channels)
self.pool = nn.AdaptiveAvgPool3d((1, 1, 1))
self.fc = nn.Linear(out_channels, 1000)
def forward(self, x):
x = self.conv(x)
for block in self.blocks:
x = block(x)
x = self.norm(x)
x = self.pool(x)
x = x.flatten(1)
x = self.fc(x)
return x
```
其中,`VideoSwinTransformerBlock`表示视频Swin Transformer的一个基本块,包含了一个注意力机制和一个多层感知机,同时使用了3D卷积来处理视频数据。`VideoSwinTransformer`则表示整个视频Swin Transformer模型,包含了多个基本块和全局平均池化层和全连接层。您可以根据自己的需求修改其中的参数和模型结构。
阅读全文
相关推荐
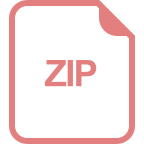
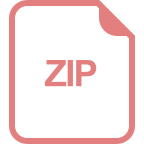
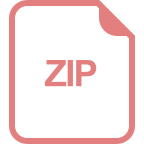
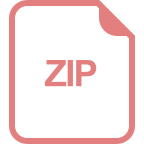
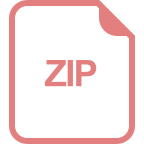
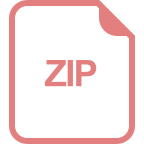
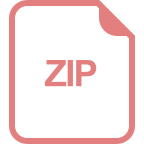
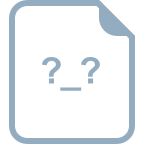