java校验时间是否为本周、上周、上个月、2023年之间
时间: 2023-10-01 10:08:40 浏览: 56
校验时间是否为本周、上周、上个月、2023年之间可以使用Java的时间处理库来实现。
首先,获取当前时间可以使用`java.util.Date`类或`java.time.LocalDateTime`类。然后,根据需求选择合适的方法进行比较。
下面是一个示例代码,用于校验时间是否为本周、上周、上个月、2023年之间:
```java
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.temporal.ChronoUnit;
public class TimeValidator {
public static boolean isThisWeek(LocalDateTime date) {
LocalDate now = LocalDate.now();
LocalDate monday = now.minusDays(now.getDayOfWeek().getValue() - 1);
LocalDate sunday = now.plusDays(7 - now.getDayOfWeek().getValue());
return date.toLocalDate().isAfter(monday.minusDays(1)) && date.toLocalDate().isBefore(sunday);
}
public static boolean isLastWeek(LocalDateTime date) {
LocalDate now = LocalDate.now();
LocalDate lastMonday = now.minusDays(now.getDayOfWeek().getValue() + 6);
LocalDate lastSunday = now.minusDays(now.getDayOfWeek().getValue());
return date.toLocalDate().isAfter(lastMonday.minusDays(1)) && date.toLocalDate().isBefore(lastSunday);
}
public static boolean isLastMonth(LocalDateTime date) {
LocalDateTime now = LocalDateTime.now();
LocalDateTime lastMonth = now.minus(1, ChronoUnit.MONTHS);
return date.isAfter(lastMonth);
}
public static boolean isYear2023(LocalDateTime date) {
LocalDateTime year2023 = LocalDateTime.of(2023, 1, 1, 0, 0, 0);
return date.isAfter(year2023);
}
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
System.out.println("Is this week: " + isThisWeek(now));
System.out.println("Is last week: " + isLastWeek(now));
System.out.println("Is last month: " + isLastMonth(now));
System.out.println("Is year 2023: " + isYear2023(now));
}
}
```
这段代码中,我们分别实现了校验时间是否为本周、上周、上个月、2023年的方法,每个方法接收一个`LocalDateTime`类型的参数,表示要校验的时间。在`main`方法中,我们获取当前时间并调用上述方法进行测试。
相关推荐
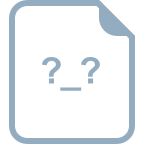
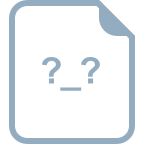














