python爱心代码
时间: 2023-09-22 21:05:43 浏览: 84
### 回答1:
这是一段使用 Python 绘制爱心的示例代码:
```python
import matplotlib.pyplot as plt
import numpy as np
t = np.linspace(0, 2*np.pi, 200)
x = 16*np.sin(t)**3
y = 13*np.cos(t)-5*np.cos(2*t)-2*np.cos(3*t)-np.cos(4*t)
plt.plot(x, y, 'r')
plt.show()
```
上面的代码使用了 matplotlib 库来绘图,numpy 库用于生成数据。其中,x 和 y 分别是爱心图形的 x 坐标和 y 坐标。'r'表示颜色为红色。
运行上面的代码可以得到一个爱心的图形,具体效果因显示器而异。
### 回答2:
Python爱心代码是指使用Python编程语言来实现绘制一个爱心的图案。下面是一个简单的Python代码示例来实现这个功能:
```python
import turtle
def draw_heart():
turtle.color('red')
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.left(120)
for i in range(200):
turtle.right(1)
turtle.forward(2)
turtle.forward(224)
turtle.end_fill()
turtle.hideturtle()
# 主程序入口
if __name__ == '__main__':
turtle.setup(800, 600)
turtle.speed(10)
turtle.penup()
turtle.goto(0, -150)
turtle.pendown()
draw_heart()
turtle.done()
```
上述代码使用了Python的turtle库来实现绘制一个爱心的图案。首先,我们定义了一个名为`draw_heart()`的函数,用于具体绘制爱心的形状。然后,在主程序入口处,我们对画布进行设置,并通过调用`draw_heart()`函数来绘制爱心图案。
运行以上代码,将会在窗口中得到一个绘制爱心的图案。这是一个简单的示例,你也可以根据自己的需求进行修改和完善。
### 回答3:
爱心代码是一种用编程语言编写的代码,以图形形式呈现出爱心的图案。Python作为一种简洁、易学的编程语言,也可以用来编写爱心代码。以下是一个简单的Python爱心代码示例:
```
import turtle
# 设置画布
screen = turtle.Screen()
screen.setup(500, 500)
screen.bgcolor("black")
# 创建画笔
pen = turtle.Turtle()
pen.shape("turtle")
pen.color("red")
pen.speed(2)
# 绘制爱心
pen.up()
pen.goto(0, -200)
pen.down()
pen.begin_fill()
pen.left(140)
pen.forward(224)
for i in range(200):
pen.right(1)
pen.forward(2)
pen.left(120)
for i in range(200):
pen.right(1)
pen.forward(2)
pen.forward(224)
pen.end_fill()
# 隐藏画笔
pen.hideturtle()
# 结束绘制
turtle.done()
```
这段代码使用了Python的turtle库来绘制爱心图案。通过在画布上绘制一系列的线段,最终呈现出一个完整的爱心形状。代码中使用了一些基本的绘图命令,如移动画笔位置、设置画笔形状和颜色、绘制线段等。运行代码后,可以看到一个红色的爱心图案显示在黑色的画布上。
虽然这只是一个简单的示例,但你可以根据个人的需求和创意,进一步扩展和美化爱心图案。编写和调试爱心代码不仅可以巩固Python编程知识,还能带来一些趣味和惊喜。希望以上回答对你有所帮助!
相关推荐
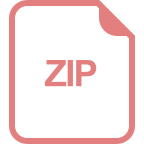
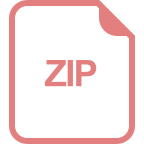
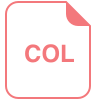
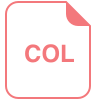
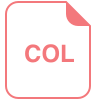
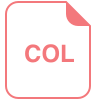
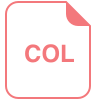






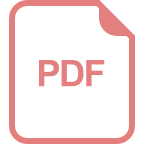
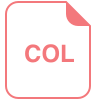
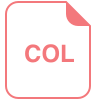