class CLine { private: int numpoint; int color; int width; vector<double,double> point; public: CLine(int NUM, int COLOR ,int WIDTH): numpoint(NUM), color(COLOR), width(WIDTH) {}; void addpoint(double x,double y){ point.push_back(make_pair(x, y)); ++numpoint; } void erasepoint(int i) { point.erase(point.begin() + i); --numpoint; } void modify(int i,int x,int y) { point[i] = make_pair(x, y); } int getnum() { return numpoint; } };这段代码有什么问题?
时间: 2023-12-13 22:02:33 浏览: 26
这段代码有以下问题:
1. 在 vector 的定义中,应该使用 `vector<pair<double,double>>` 而不是 `vector<double,double>`。
2. 在 `modify` 函数中,应该传入两个 double 类型的参数,而不是两个 int 类型的参数。
3. 在 `modify` 函数中,应该使用 `point[i] = make_pair(x, y);` 而不是 `point[i] = make_pair(int(x), int(y));`。
4. 在构造函数中,没有初始化 point 变量,可能会导致未定义行为。
5. 如果使用 `erase` 函数删除最后一个点,会导致程序崩溃,因为不能删除空的 vector。
6. 在 `addpoint` 函数中,应该先添加点,再将 numpoint 自增,否则会出现错误的点数。
以下是修改后的代码:
```
class CLine {
private:
int numpoint;
int color;
int width;
vector<pair<double,double>> point;
public:
CLine(int NUM, int COLOR ,int WIDTH): numpoint(NUM), color(COLOR), width(WIDTH), point() {};
void addpoint(double x,double y){
point.push_back(make_pair(x, y));
++numpoint;
}
void erasepoint(int i) {
if (!point.empty()) {
point.erase(point.begin() + i);
--numpoint;
}
}
void modify(int i,double x,double y) {
point[i] = make_pair(x, y);
}
int getnum() { return numpoint; }
};
```
相关推荐
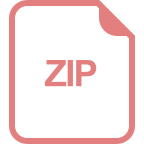
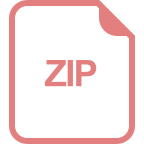
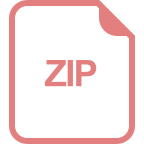














