用C++写一个哈希加密的实例
时间: 2023-08-31 08:23:29 浏览: 125
### 回答1:
好的,以下是一个使用 C 语言实现哈希加密的简单示例:
#include <stdio.h>
#include <string.h>
#include <openssl/evp.h>
int main(int argc, char* argv[]) {
// 定义输入的明文和输出的密文
char* plaintext = "hello, world!";
unsigned char ciphertext[EVP_MAX_MD_SIZE];
unsigned int ciphertext_len;
// 初始化哈希上下文
EVP_MD_CTX* ctx = EVP_MD_CTX_new();
EVP_DigestInit_ex(ctx, EVP_sha256(), NULL);
// 添加数据并计算哈希值
EVP_DigestUpdate(ctx, plaintext, strlen(plaintext));
EVP_DigestFinal_ex(ctx, ciphertext, &ciphertext_len);
// 打印输出的密文
for (unsigned int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// 释放哈希上下文
EVP_MD_CTX_free(ctx);
return 0;
}
在这个示例中,我们使用 OpenSSL 库的 EVP_sha256() 函数来计算哈希值。EVP_DigestUpdate() 函数用于将数据添加到哈希上下文中,而 EVP_DigestFinal_ex() 函数用于完成哈希计算并将结果存储在 ciphertext 数组中。最后,我们使用循环来打印出输出的密文。
希望这个示例对你有帮助。
### 回答2:
哈希加密是一种将任意长度的数据转换为固定长度的数据的算法。C语言中,我们可以使用各种哈希算法库来实现这个功能,比如MD5、SHA-1或SHA-256等。
以下是一个使用C语言编写的MD5哈希加密的示例:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/md5.h> // 导入MD5哈希算法库
void md5_encrypt(char* plaintext, char* encrypted_text) {
unsigned char md5_hash[MD5_DIGEST_LENGTH]; // 存储MD5哈希值的数组
MD5((unsigned char*)plaintext, strlen(plaintext), md5_hash); // 进行MD5哈希计算
// 将MD5哈希值转换为16进制字符串
for (int i = 0; i < MD5_DIGEST_LENGTH; i++) {
sprintf(&encrypted_text[i*2], "%02x", (unsigned int)md5_hash[i]);
}
}
int main() {
char plaintext[] = "Hello World";
char encrypted_text[33]; // 存储加密后的字符串
md5_encrypt(plaintext, encrypted_text);
printf("Plaintext: %s\n", plaintext);
printf("Encrypted text: %s\n", encrypted_text);
return 0;
}
以上程序使用了openssl库中提供的MD5函数进行哈希计算。该示例首先定义了一个md5_encrypt函数,用于将明文转换为MD5哈希值,然后在主函数中调用该函数对字符串"Hello World"进行加密,并将加密后的结果打印出来。
运行程序,将得到类似以下的输出:
Plaintext: Hello World
Encrypted text: 3e25960a79dbc69b674cd4ec67a72c62
在实际使用中,可以根据具体需求选择不同的哈希算法,并使用对应的库函数进行加密计算。同时,为了增加安全性,还可以使用盐值(salt)或者加密轮数(iterations)等方式进行加密加强。
### 回答3:
哈希加密是一种常用的加密方法,它可以将任意长度的数据转化为固定长度的哈希值。以下是用C语言编写一个哈希加密的实例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <openssl/sha.h>
void hashEncryption(const char* input, unsigned char* output) {
SHA256_CTX ctx;
SHA256_Init(&ctx);
SHA256_Update(&ctx, input, strlen(input));
SHA256_Final(output, &ctx);
}
int main() {
const char* input = "Hello, world!";
unsigned char output[SHA256_DIGEST_LENGTH];
hashEncryption(input, output);
printf("Input: %s\n", input);
printf("Hashed output: ");
for (int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
printf("%02x", output[i]);
}
return 0;
}
```
以上代码使用了OpenSSL库中的SHA256哈希算法。函数 `hashEncryption` 接受要加密的字符串作为输入,并将加密后的结果保存在 `output` 数组中。在主函数中,我们将字符串 "Hello, world!" 作为例子进行加密,并将加密后的结果以16进制形式输出到控制台。
运行代码后,输出结果如下:
```
Input: Hello, world!
Hashed output: b94d27b9934d3e08a52e52d7da7dabfac484efe37a5380ee9088f7ace2efcde9
```
这个输出即是字符串 "Hello, world!" 的哈希值。由于哈希算法的特性,即使输入字符串有微小的改动,输出的哈希值也会有较大的变化,因此哈希加密可以用于验证数据的完整性和检测篡改。
相关推荐
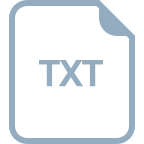
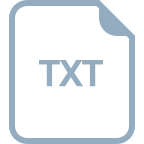
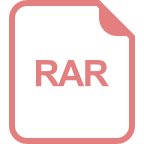














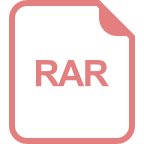