c#如何连接数据库
时间: 2023-07-03 16:06:38 浏览: 59
在C#中连接数据库,需要使用ADO.NET技术。以下是连接数据库的基本步骤:
1. 导入命名空间:使用System.Data.SqlClient命名空间。
2. 创建SqlConnection对象:用于与数据库建立连接。
3. 打开数据库连接:使用SqlConnection对象的Open()方法。
4. 创建SqlCommand对象:用于执行SQL语句。
5. 执行SQL语句:使用SqlCommand对象的ExecuteNonQuery()方法(用于执行增删改操作)或ExecuteReader()方法(用于执行查询操作)。
6. 关闭数据库连接:使用SqlConnection对象的Close()方法。
下面是一个简单的示例代码,连接到名为“mydb”的数据库,并查询其中的“employees”表:
```csharp
using System.Data.SqlClient;
string connectionString = "Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;";
SqlConnection connection = new SqlConnection(connectionString);
try
{
connection.Open();
SqlCommand command = new SqlCommand("SELECT * FROM employees", connection);
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine(reader["employee_id"].ToString() + " - " + reader["employee_name"].ToString());
}
reader.Close();
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
finally
{
connection.Close();
}
```
需要注意的是,连接字符串中的“myServerAddress”应该替换为实际的服务器地址,“myDataBase”应该替换为实际的数据库名称,“myUsername”和“myPassword”应该分别替换为实际的用户名和密码。
相关推荐
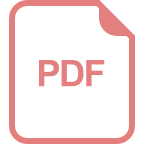















