使用vue3写一个表格传参示例,使用axios传参
时间: 2023-07-20 12:31:10 浏览: 46
好的,下面是一个使用 Vue 3 和 Axios 发起 HTTP 请求来获取表格数据的示例:
```vue
<template>
<div>
<h2>{{ tableName }}</h2>
<table>
<thead>
<tr>
<th v-for="header in headers" :key="header">{{ header }}</th>
</tr>
</thead>
<tbody>
<tr v-for="row in rows" :key="row.id">
<td v-for="column in columns" :key="column">{{ row[column] }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import axios from "axios";
export default {
props: {
tableName: {
type: String,
required: true
},
headers: {
type: Array,
required: true
},
url: {
type: String,
required: true
},
params: {
type: Object,
default: () => ({})
}
},
data() {
return {
rows: []
};
},
computed: {
columns() {
// 获取所有列名
return Object.keys(this.rows[0] || {});
}
},
async created() {
try {
const response = await axios.get(this.url, { params: this.params });
this.rows = response.data;
} catch (error) {
console.error(error);
}
}
};
</script>
```
在父组件中,可以这样使用这个表格组件:
```vue
<template>
<div>
<my-table
:table-name="'用户列表'"
:headers="['ID', '姓名', '年龄']"
url="/api/users"
:params="{ page: 1, pageSize: 10 }"
/>
</div>
</template>
<script>
import MyTable from "@/components/MyTable.vue";
export default {
components: {
MyTable
}
};
</script>
```
这样就可以在页面中显示一个名为“用户列表”的表格,表格的表头为“ID”、“姓名”、“年龄”,表格的数据来自服务器端的 `/api/users` 接口,请求参数为 `{ page: 1, pageSize: 10 }`。
相关推荐
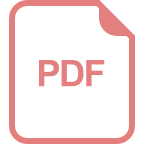














