mybatisplus的ipage分页代码写一下
时间: 2023-03-22 14:01:19 浏览: 71
很高兴为您解答,MybatisPlus中的IPage分页代码如下:
IPage<T> page = new Page<>(1, 10);
IPage<T> result = myTableService.page(page);
相关问题
mybatisplus的ipage分页
Mybatis Plus提供了IPage分页功能,它可以方便地实现数据库分页。它实现了Page接口,并提供了一些常用的分页方法。使用IPage可以简化分页查询的代码,并且可以在Mybatis Plus的Mapper接口中直接使用。
mybatisplus分页查询代码
以下是Mybatis-plus的分页查询代码:
<<引用:完整配置类代码>>
```
@Configuration
public class MybatisPlusConfig {
/**
* 分页插件
*/
@Bean
public PaginationInterceptor paginationInterceptor() {
return new PaginationInterceptor();
}
}
```
<<引用:示例代码>>
```
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public IPage<User> getUserList(Page<User> page) {
return userMapper.selectPage(page, null);
}
}
```
在上述示例代码中,我们使用了`selectPage`方法来进行分页查询,其中`page`参数是`Page`类型,表示分页查询的条件,`null`表示没有查询条件。在`getUserList`方法中,我们将查询结果封装成`IPage<User>`类型并返回。
需要注意的是,在获取分页查询结果时,我们可以使用`page.getRecords()`方法来获取查询出来的数据。
相关推荐
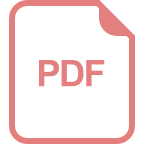
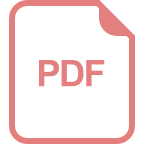












