ISO 15693协议读取指定块的命令是什么
时间: 2023-12-21 17:05:38 浏览: 230
ISO 15693协议读取指定块的命令是读块命令,其指令代码为0x20。读块命令的具体格式为:
```
Flags | Command | Block number
-------------------------------
1 byte | 1 byte | 1 byte
```
其中Flags为标志位,Command为指令代码,Block number为要读取的块号。根据协议规定,Block number的取值范围为0x00~0xFF。因此,读取指定块的命令可以表示为:
```
byte[] readCmd = new byte[] { (byte) 0x20, (byte) blockNumber };
```
其中blockNumber为要读取的块号。
相关问题
如何读取ISO15693 标签指定块
要读取 ISO15693 标签的指定块,需要遵循 ISO15693 标准协议,具体步骤如下:
1. 连接 RFID 标签,获取 `NfcV` 对象
```
NfcV nfcv = NfcV.get(tag);
nfcv.connect();
```
2. 发送 `READ_SINGLE_BLOCK` 命令读取指定块的数据。命令格式为:`0x20 0x20 0xYY`,其中 `YY` 为指定块的块号(十六进制表示)
```
// 假设要读取第 2 块的数据
byte[] readCmd = new byte[] { (byte) 0x20, (byte) 0x20, (byte) 0x02 };
byte[] data = nfcv.transceive(readCmd);
```
3. 处理读取到的数据。如果读取成功,返回的数据应该是指定块的数据。如果读取失败,可能会返回错误码等信息
```
if (data != null && data.length >= 1 && data[0] == 0x00) {
// 读取成功,获取数据
byte[] blockData = new byte[4];
System.arraycopy(data, 1, blockData, 0, 4);
// 处理读取到的数据
...
} else {
// 读取失败,处理错误码等信息
...
}
```
4. 关闭连接,释放资源
```
nfcv.close();
```
完整的读取指定块的代码示例如下:
```
import android.nfc.NfcAdapter;
import android.nfc.Tag;
import android.nfc.tech.NfcV;
import java.io.IOException;
public class Iso15693Reader {
public static byte[] readBlock(Tag tag, int blockNumber) throws IOException {
NfcV nfcv = NfcV.get(tag);
nfcv.connect();
byte[] readCmd = new byte[] { (byte) 0x20, (byte) 0x20, (byte) blockNumber };
byte[] data = nfcv.transceive(readCmd);
nfcv.close();
if (data != null && data.length >= 1 && data[0] == 0x00) {
byte[] blockData = new byte[4];
System.arraycopy(data, 1, blockData, 0, 4);
return blockData;
} else {
throw new IOException("读取块失败");
}
}
}
```
使用时,可以调用 `Iso15693Reader.readBlock(tag, blockNumber)` 方法读取指定块的数据,其中 `tag` 是 `Tag` 对象,`blockNumber` 是指定块的块号。如果读取成功,返回的是指定块的数据;如果读取失败,会抛出 `IOException` 异常。
阅读全文
相关推荐
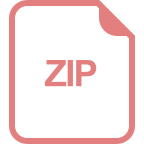
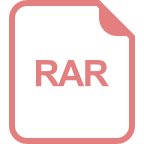
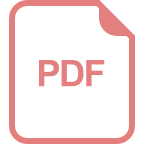
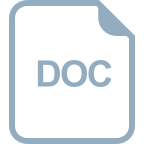
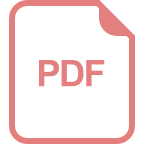
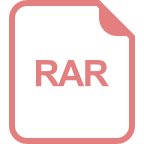
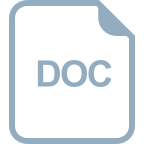
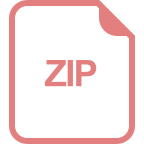
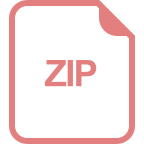
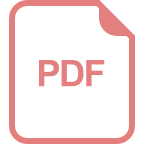
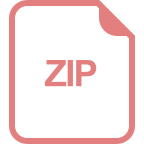
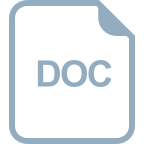
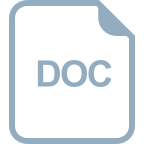
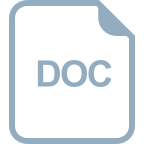
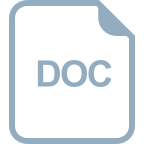
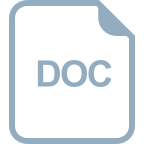
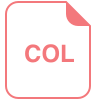
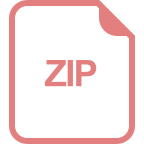