设置listwidget点击空白处取消选中
时间: 2023-10-08 19:08:58 浏览: 437
您可以使用ListWidget的setSelectionMode方法来设置选择模式为单选(QAbstractItemView.SingleSelection),然后在ListWidget的mousePressEvent事件处理程序中检查是否单击了空白处来取消选择项,示例如下:
```python
from PyQt5.QtWidgets import QListWidget
from PyQt5.QtCore import Qt
class MyListWidget(QListWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.setSelectionMode(QAbstractItemView.SingleSelection)
def mousePressEvent(self, event):
index = self.indexAt(event.pos())
if not index.isValid():
self.clearSelection()
else:
super().mousePressEvent(event)
```
这样,当用户单击ListWidget的空白处时,当前选择的项将被取消选择。
相关问题
pyqt6 listwidget 点击空白处取消选中项
在PyQt6中,`QListWidget`是一个用于显示列表的控件,如果你想让用户点击空白区域来取消选中的项目,可以利用`QAbstractItemView`的行为设置。`QListWidget`默认不会响应空白区域的单击事件,但你可以通过继承并覆盖其内置的一些槽函数来实现这个功能。
首先,你需要创建自定义的`MyListWidget`类,继承自`QListWidget`:
```python
from PyQt6.QtWidgets import QListWidget, QWidget
class MyListWidget(QListWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.setMouseTracking(True) # 允许鼠标追踪
def mouseReleaseEvent(self, event): # 当鼠标释放时触发
if not self.hasFocus() and event.button() == Qt.LeftButton:
self.clearSelection() # 如果不在焦点内且左键点击空白,清空选择
```
然后,在你的应用中实例化并使用这个新的`MyListWidget`:
```python
app = QApplication([])
main_widget = QWidget()
list_view = MyListWidget(main_widget)
# ...其他布局设置...
main_widget.show()
app.exec_()
```
现在,当用户在空白区域左键点击时,`MyListWidget`会自动清除已选中的项目。如果你还有其他疑问,例如如何处理滚动时的空白点击,可以在`mouseMoveEvent`或`wheelEvent`等函数中添加相应的逻辑。
如何通过c++ qt中的listwideget的itemselectionchanged信号实现单击选中且点击空白处取消选中
您可以通过以下代码实现您所需的功能:
```
// 连接 listwidget 的 itemSelectionChanged 信号到槽函数
connect(ui->listWidget, SIGNAL(itemSelectionChanged()), this, SLOT(onListWidgetItemSelectionChanged()));
// 实现槽函数
void YourClass::onListWidgetItemSelectionChanged() {
// 获取选中的 item
QList<QListWidgetItem *> selectedItems = ui->listWidget->selectedItems();
// 如果没有任何选中的 item,则清除之前的选中状态
if (selectedItems.isEmpty()) {
ui->listWidget->setCurrentItem(nullptr);
}
// 如果有选中的 item,则将其设置为当前 item
// 注意:此处只支持单选,如果您需要多选,则需要调整代码逻辑
else {
ui->listWidget->setCurrentItem(selectedItems.first());
}
}
```
以上代码中,我们先将 listwidget 的 itemSelectionChanged 信号连接到一个槽函数(这里我们使用了 onListWidgetItemSelectionChanged 函数)。在槽函数中,我们首先获取当前选中的 item(如果没有选中任何 item,则 selectedItems 为一个空列表)。如果没有选中任何 item,则将当前 item 设置为 nullptr,即清除之前的选中状态;否则,将选中的第一个 item 设置为当前 item。
需要注意的是,以上代码只支持单选,如果您需要多选,则需要根据实际需要调整代码逻辑。
阅读全文
相关推荐
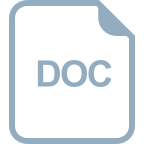







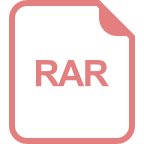
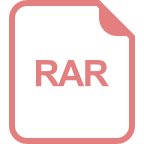
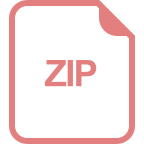
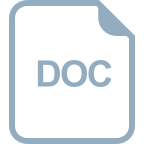
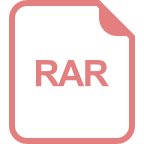