springboot整合微信公众号
时间: 2023-10-01 19:07:28 浏览: 125
Spring Boot 可以很方便地与微信公众号进行整合。下面是一个 Spring Boot 整合微信公众号的简单步骤:
1.创建一个微信公众号并获取 AppID 和 AppSecret。
2.在 pom.xml 中添加以下依赖:
```
<dependency>
<groupId>com.github.sd4324530</groupId>
<artifactId>weixin-java-tools-spring-boot-starter</artifactId>
<version>2.9.0</version>
</dependency>
```
3.在 application.properties 中配置 AppID 和 AppSecret:
```
wechat.appId=yourAppId
wechat.appSecret=yourAppSecret
wechat.token=yourToken
wechat.aesKey=yourAesKey
```
4.创建一个类来配置微信公众号:
```
@Configuration
public class WechatConfig {
@Autowired
private WechatProperties wechatProperties;
@Bean
public WxMpConfigStorage wxMpConfigStorage(){
WxMpInMemoryConfigStorage configStorage = new WxMpInMemoryConfigStorage();
configStorage.setAppId(wechatProperties.getAppId());
configStorage.setSecret(wechatProperties.getAppSecret());
configStorage.setToken(wechatProperties.getToken());
configStorage.setAesKey(wechatProperties.getAesKey());
return configStorage;
}
@Bean
public WxMpService wxMpService(WxMpConfigStorage wxMpConfigStorage){
WxMpService wxMpService = new WxMpServiceImpl();
wxMpService.setWxMpConfigStorage(wxMpConfigStorage);
return wxMpService;
}
}
```
5.在 Controller 中处理微信公众号的消息:
```
@RestController
@RequestMapping("/wechat")
public class WechatController {
@Autowired
private WxMpService wxMpService;
@GetMapping(produces = "text/plain;charset=utf-8")
public String authGet(@RequestParam(name = "signature", required = false) String signature,
@RequestParam(name = "timestamp", required = false) String timestamp,
@RequestParam(name = "nonce", required = false) String nonce,
@RequestParam(name = "echostr", required = false) String echostr) {
if (!wxMpService.checkSignature(timestamp, nonce, signature)) {
return "非法请求";
}
return echostr;
}
@PostMapping(produces = "application/xml; charset=UTF-8")
public String post(@RequestBody String requestBody,
@RequestParam("signature") String signature,
@RequestParam("timestamp") String timestamp,
@RequestParam("nonce") String nonce,
@RequestParam("openid") String openid,
@RequestParam(name = "encrypt_type", required = false) String encType,
@RequestParam(name = "msg_signature", required = false) String msgSignature) {
if (!wxMpService.checkSignature(timestamp, nonce, signature)) {
throw new IllegalArgumentException("非法请求,可能属于伪造的请求!");
}
WxMpXmlMessage wxMessage;
if (encType == null) {
wxMessage = WxMpXmlMessage.fromXml(requestBody);
} else {
wxMessage = WxMpXmlMessage.fromEncryptedXml(requestBody, wxMpService.getWxMpConfigStorage(), timestamp, nonce, msgSignature);
}
String content = "收到消息内容:" + wxMessage.getContent();
WxMpXmlOutMessage outMessage = WxMpXmlOutMessage.TEXT().content(content).fromUser(wxMessage.getToUser())
.toUser(wxMessage.getFromUser()).build();
return outMessage.toXml();
}
}
```
现在你就可以通过该 Controller 处理微信公众号的消息了。
阅读全文
相关推荐
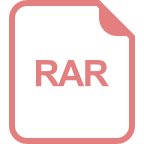
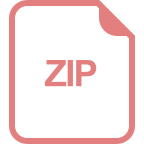















