用C++NurHar: 设计一个Bank类,实现银行某账户资金往来账目管理。程序要求完成以下操作。 NurHar: 1, 创建账户:包括账号,创建日期,余额(创建账户时存入的钱数) NurHar: 2存钱:执行存钱操作,并记录存钱日期和存钱数目 NurHar: 3,取钱:执行取钱操作,并记录取钱日期和取钱数目。 NurHar: 4,查询交易明细:查询近一个月的账户交易记录
时间: 2024-03-25 09:40:43 浏览: 95
好的,下面是Bank类的设计及实现代码:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <ctime>
using namespace std;
class Account {
public:
Account(string number, double balance) : number(number), balance(balance) {
time_t now = time(nullptr);
created = ctime(&now);
}
void deposit(double amount) {
balance += amount;
time_t now = time(nullptr);
string date = ctime(&now);
transactions.push_back("Deposit of " + to_string(amount) + " on " + date);
}
void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
time_t now = time(nullptr);
string date = ctime(&now);
transactions.push_back("Withdrawal of " + to_string(amount) + " on " + date);
} else {
cout << "Insufficient balance!" << endl;
}
}
void showTransactions() {
time_t now = time(nullptr);
string endDate = ctime(&now);
string startDate = ctime(&now - 30 * 24 * 60 * 60);
cout << "Transactions from " << startDate << "to " << endDate << endl;
for (auto transaction : transactions) {
if (transaction.compare(11, 24, startDate) >= 0 && transaction.compare(11, 24, endDate) <= 0) {
cout << transaction << endl;
}
}
}
void showDetails() {
cout << "Account number: " << number << endl;
cout << "Created on: " << created;
cout << "Balance: " << balance << endl;
}
private:
string number;
string created;
double balance;
vector<string> transactions;
};
class Bank {
public:
void createAccount() {
string number;
double balance;
cout << "Enter account number: ";
cin >> number;
cout << "Enter initial balance: ";
cin >> balance;
Account account(number, balance);
accounts.push_back(account);
cout << "Account created successfully!" << endl;
}
void deposit() {
string number;
double amount;
cout << "Enter account number: ";
cin >> number;
cout << "Enter amount to deposit: ";
cin >> amount;
for (auto& account : accounts) {
if (account.number == number) {
account.deposit(amount);
cout << "Deposit successful!" << endl;
return;
}
}
cout << "Account not found!" << endl;
}
void withdraw() {
string number;
double amount;
cout << "Enter account number: ";
cin >> number;
cout << "Enter amount to withdraw: ";
cin >> amount;
for (auto& account : accounts) {
if (account.number == number) {
account.withdraw(amount);
return;
}
}
cout << "Account not found!" << endl;
}
void showTransactions() {
string number;
cout << "Enter account number: ";
cin >> number;
for (auto& account : accounts) {
if (account.number == number) {
account.showTransactions();
return;
}
}
cout << "Account not found!" << endl;
}
void showDetails() {
string number;
cout << "Enter account number: ";
cin >> number;
for (auto& account : accounts) {
if (account.number == number) {
account.showDetails();
return;
}
}
cout << "Account not found!" << endl;
}
private:
vector<Account> accounts;
};
int main() {
Bank bank;
int choice;
while (true) {
cout << "1. Create account" << endl;
cout << "2. Deposit" << endl;
cout << "3. Withdraw" << endl;
cout << "4. Show transactions" << endl;
cout << "5. Show details" << endl;
cout << "6. Exit" << endl;
cout << "Enter choice: ";
cin >> choice;
switch (choice) {
case 1:
bank.createAccount();
break;
case 2:
bank.deposit();
break;
case 3:
bank.withdraw();
break;
case 4:
bank.showTransactions();
break;
case 5:
bank.showDetails();
break;
case 6:
return 0;
default:
cout << "Invalid choice!" << endl;
}
}
}
```
Bank类包含了创建账户、存钱、取钱、查询交易明细、查询账户详情等操作。每个账户有一个账号、创建日期、余额和交易记录,交易记录包含了交易日期、交易类型和交易金额。当执行存钱或取钱操作时,会记录一条交易记录。查询交易明细时,会按照交易日期筛选出近一个月的交易记录并输出。查询账户详情时,会输出账户的账号、创建日期和余额。
在main函数中,我们创建了一个Bank对象,并提供了一个简单的菜单供用户选择操作。用户可以输入数字来选择相应的操作,或输入6来退出程序。
阅读全文
相关推荐


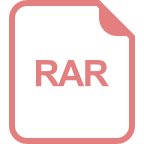

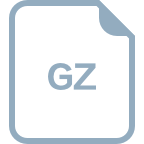




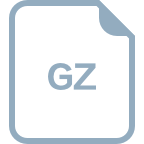
