epoll线程池服务器设计
时间: 2024-05-30 07:07:12 浏览: 31
epoll线程池服务器是一种高性能的服务器设计模式,它主要使用了Linux内核提供的epoll机制和线程池技术,可以实现高并发、高吞吐量的网络服务器。
具体来说,epoll线程池服务器的设计大致包括以下几个步骤:
1. 创建监听socket,并将其加入到epoll事件集中。
2. 使用epoll_wait函数阻塞等待事件的发生,一旦有新的连接请求到来,epoll_wait会返回并将该事件加入到epoll事件集中。
3. 当有新的连接请求到来时,服务器会从线程池中取出一个线程进行处理,处理完毕后将该线程放回线程池。
4. 在每个线程中,使用epoll机制监听来自客户端的消息,并根据消息类型进行处理。
5. 当客户端关闭连接时,服务器会将该连接从epoll事件集中删除,并释放相关资源。
相关问题
epoll线程池实现高并发服务器c++11
以下是使用epoll和线程池实现高并发服务器的C++11代码示例:
```cpp
#include <iostream>
#include <thread>
#include <vector>
#include <queue>
#include <mutex>
#include <condition_variable>
#include <sys/epoll.h>
#include <unistd.h>
#define MAX_EVENTS 100
#define THREAD_POOL_SIZE 10
std::mutex mtx;
std::condition_variable cv;
std::queue<int> taskQueue;
void workerThread()
{
while (true)
{
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !taskQueue.empty(); });
int fd = taskQueue.front();
taskQueue.pop();
// 处理任务,这里可以根据具体需求进行处理
lock.unlock();
// 继续监听其他事件
}
}
int main()
{
// 创建epoll句柄
int epoll_fd = epoll_create(1);
if (epoll_fd == -1)
{
std::cerr << "Failed to create epoll" << std::endl;
return 1;
}
// 创建线程池
std::vector<std::thread> threadPool;
for (int i = 0; i < THREAD_POOL_SIZE; ++i)
{
threadPool.emplace_back(workerThread);
}
// 添加监听事件到epoll句柄
struct epoll_event event;
event.events = EPOLLIN;
event.data.fd = /* 监听的文件描述符 */;
if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, /* 监听的文件描述符 */, &event) == -1)
{
std::cerr << "Failed to add event to epoll" << std::endl;
return 1;
}
// 开始监听事件
struct epoll_event events[MAX_EVENTS];
while (true)
{
int num_events = epoll_wait(epoll_fd, events, MAX_EVENTS, -1);
if (num_events == -1)
{
std::cerr << "Failed to wait for events" << std::endl;
return 1;
}
for (int i = 0; i < num_events; ++i)
{
if (events[i].events & EPOLLIN)
{
// 处理读事件,将任务添加到任务队列
std::lock_guard<std::mutex> lock(mtx);
taskQueue.push(events[i].data.fd);
cv.notify_one();
}
}
}
// 清理资源
close(epoll_fd);
for (auto& thread : threadPool)
{
thread.join();
}
return 0;
}
```
EPOLL和线程池搭配使用C++
EPOLL和线程池是在C++中常用的技术,用于实现高效的网络编程。EPOLL是Linux下的一种I/O多路复用机制,可以同时监控多个文件描述符的状态变化,从而实现高并发的网络通信。线程池则是一种线程管理机制,通过预先创建一定数量的线程,将任务分配给这些线程来执行,从而提高程序的并发性能。
在C++中,可以使用EPOLL和线程池来实现高性能的网络服务器。EPOLL负责监听和处理网络事件,而线程池则负责处理具体的业务逻辑。当有新的连接到来时,EPOLL会将该连接的文件描述符添加到监听列表中,并在有事件发生时通知线程池进行处理。线程池中的线程会从任务队列中取出任务,并执行相应的操作。通过EPOLL和线程池的搭配使用,可以实现高并发的网络通信,并提高程序的性能和可扩展性。
关于EPOLL和线程池的具体实现细节,可以参考相关的书籍和文章。例如,可以阅读《C++ Primer》和《C++ Concurrency in Action》等C++基础书籍,了解EPOLL和线程池的基本原理和用法。此外,还可以参考一些经验分享的文章,如CSDN上的《Visual Studio高效调试手段与技巧总结》和《C++没落了?学习C++没有前途了?从业者给你揭晓答案》等,获取更多关于EPOLL和线程池的实践经验和技巧。\[1\]\[2\]\[3\]
#### 引用[.reference_title]
- *1* *2* *3* [C/C++学习路线总结与分享](https://blog.csdn.net/chenlycly/article/details/127191075)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
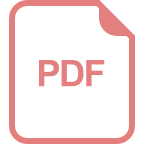
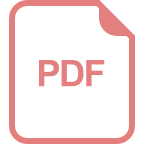
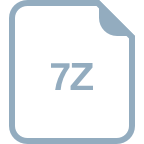












