java项目通过mybatis实现多数据源实例
时间: 2023-10-13 13:28:12 浏览: 41
在Java项目中,通过MyBatis实现多数据源实例可以按照以下步骤进行:
1. 在项目中引入MyBatis和数据库驱动。
2. 在项目中定义多个数据源,对应不同的数据库。可以使用Spring框架的`DataSource`接口实现类来定义数据源。
3. 配置MyBatis的SqlSessionFactory,指定多个数据源。
4. 在Mapper接口中使用`@MapperScan`注解指定要扫描的Mapper接口路径。
5. 在Mapper.xml文件中,使用`<selectKey>`标签指定使用的数据源。
示例代码如下:
```
//定义数据源1
@Bean
@ConfigurationProperties(prefix = "spring.datasource.db1")
public DataSource dataSource1() {
return DataSourceBuilder.create().build();
}
//定义数据源2
@Bean
@ConfigurationProperties(prefix = "spring.datasource.db2")
public DataSource dataSource2() {
return DataSourceBuilder.create().build();
}
//配置MyBatis的SqlSessionFactory,指定多个数据源
@Bean
public SqlSessionFactory sqlSessionFactory(@Qualifier("dataSource1") DataSource dataSource1,
@Qualifier("dataSource2") DataSource dataSource2) throws Exception {
SqlSessionFactoryBean sqlSessionFactoryBean = new SqlSessionFactoryBean();
sqlSessionFactoryBean.setDataSource(dataSource1);
//设置Mapper.xml文件路径
Resource[] mapperLocations = new PathMatchingResourcePatternResolver().getResources("classpath*:mapping/*.xml");
sqlSessionFactoryBean.setMapperLocations(mapperLocations);
//设置多个数据源
Map<Object, Object> targetDataSources = new HashMap<>();
targetDataSources.put("dataSource1", dataSource1);
targetDataSources.put("dataSource2", dataSource2);
DynamicDataSource dataSource = new DynamicDataSource();
dataSource.setTargetDataSources(targetDataSources);
dataSource.setDefaultTargetDataSource(dataSource1);
sqlSessionFactoryBean.setDataSource(dataSource);
return sqlSessionFactoryBean.getObject();
}
//在Mapper.xml文件中,使用<selectKey>标签指定使用的数据源
<select id="getUserInfoById" resultMap="userInfo" >
<selectKey resultType="java.lang.Integer" order="BEFORE" keyProperty="dataSource">
SELECT
CASE
WHEN id < 1000 THEN 'dataSource1'
ELSE 'dataSource2'
END AS dataSource
FROM user_info
WHERE id = #{id}
</selectKey>
SELECT * FROM user_info WHERE id = #{id}
</select>
```
其中,我们使用了`DynamicDataSource`类来实现动态数据源的切换。在`DynamicDataSource`类中,我们需要重写`determineCurrentLookupKey()`方法,根据具体的业务场景来动态切换数据源。
```
public class DynamicDataSource extends AbstractRoutingDataSource {
@Override
protected Object determineCurrentLookupKey() {
return DataSourceContextHolder.getDataSourceType();
}
}
```
最后,我们需要在业务代码中设置数据源的类型,以实现动态切换数据源。
```
public class UserServiceImpl implements UserService {
@Override
public UserInfo getUserInfoById(Integer id) {
DataSourceContextHolder.setDataSourceType("dataSource1");
UserInfo userInfo = userMapper.getUserInfoById(id);
return userInfo;
}
}
```
以上就是通过MyBatis实现多数据源实例的基本步骤。
相关推荐
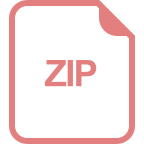
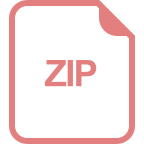














