完成一个实现秒表功能的Applet程序
时间: 2024-02-28 18:55:10 浏览: 80
好的,以下是一个实现秒表功能的Applet程序的示例代码:
```java
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
public class Stopwatch extends Applet implements Runnable, ActionListener {
// 定义按钮和标签
Button startButton, stopButton, resetButton;
Label timeLabel;
// 定义线程和计时器变量
Thread timerThread;
int timeCounter;
// 初始化Applet界面
public void init() {
// 设置界面布局
setLayout(new BorderLayout());
// 创建按钮和标签
startButton = new Button("Start");
stopButton = new Button("Stop");
resetButton = new Button("Reset");
timeLabel = new Label("00:00:00");
// 添加按钮和标签
add(timeLabel, BorderLayout.CENTER);
Panel buttonPanel = new Panel();
buttonPanel.add(startButton);
buttonPanel.add(stopButton);
buttonPanel.add(resetButton);
add(buttonPanel, BorderLayout.SOUTH);
// 添加按钮事件监听器
startButton.addActionListener(this);
stopButton.addActionListener(this);
resetButton.addActionListener(this);
}
// 实现按钮事件处理
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
// 启动线程
if (timerThread == null) {
timerThread = new Thread(this);
timerThread.start();
}
} else if (e.getSource() == stopButton) {
// 停止线程
if (timerThread != null) {
timerThread.interrupt();
timerThread = null;
}
} else if (e.getSource() == resetButton) {
// 重置计时器
timeCounter = 0;
updateTimeLabel();
}
}
// 实现线程运行
public void run() {
try {
while (true) {
Thread.sleep(1000);
timeCounter++;
updateTimeLabel();
}
} catch (InterruptedException e) {
// 线程被中断,忽略异常
}
}
// 更新时间标签
private void updateTimeLabel() {
int hours = timeCounter / 3600;
int minutes = (timeCounter % 3600) / 60;
int seconds = timeCounter % 60;
timeLabel.setText(String.format("%02d:%02d:%02d", hours, minutes, seconds));
}
}
```
该程序使用了Applet界面,包含了三个按钮和一个标签。通过点击“Start”按钮启动计时器线程,通过点击“Stop”按钮停止计时器线程,通过点击“Reset”按钮重置计时器。计时器的时间以“小时:分钟:秒”格式显示在标签中。
注意:由于Java Applet已经被弃用,该示例代码可能无法在最新的浏览器中正常运行。建议使用JavaFX或Swing等替代方案。
阅读全文
相关推荐
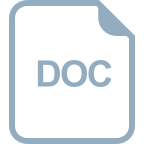
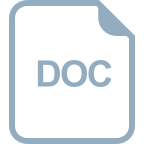
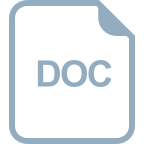
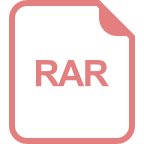
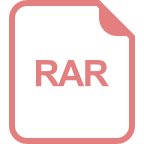
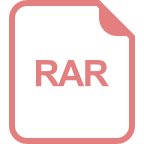
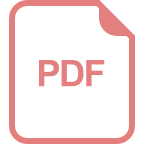
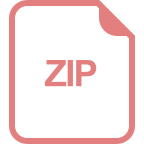
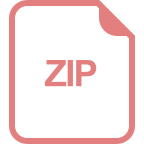
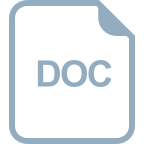
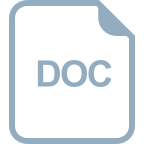
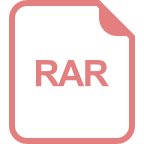
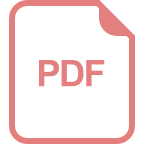
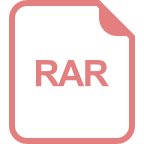
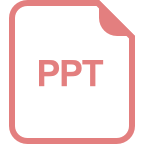