写一段代码,在Androidstudio中解析本地.geojson文件
时间: 2024-05-01 19:23:20 浏览: 20
可以使用Android中的GSON库来解析GeoJSON文件。下面是一个简单的示例代码:
1. 首先在build.gradle文件中添加GSON库的依赖:
```
dependencies {
implementation 'com.google.code.gson:gson:2.8.6'
}
```
2. 创建一个类来表示GeoJSON中的要素:
```
public class GeoJsonFeature {
private String type;
private Geometry geometry;
public String getType() {
return type;
}
public Geometry getGeometry() {
return geometry;
}
public static class Geometry {
private String type;
private List<List<Double>> coordinates;
public String getType() {
return type;
}
public List<List<Double>> getCoordinates() {
return coordinates;
}
}
}
```
3. 在Activity中读取并解析GeoJSON文件:
```
try {
// 读取GeoJSON文件
InputStream inputStream = getResources().openRawResource(R.raw.geojson_file);
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder stringBuilder = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
}
reader.close();
String geoJsonString = stringBuilder.toString();
// 解析GeoJSON文件
Gson gson = new Gson();
GeoJsonFeature feature = gson.fromJson(geoJsonString, GeoJsonFeature.class);
// 获取要素的类型和坐标
String type = feature.getType();
List<List<Double>> coordinates = feature.getGeometry().getCoordinates();
// 处理要素
// ...
} catch (IOException e) {
e.printStackTrace();
}
```
以上代码可以读取并解析一个名为"geojson_file.geojson"的文件,该文件应位于res/raw目录下。注意,这里使用了GSON库的fromJson方法来解析GeoJSON文件,并将其转换为GeoJsonFeature对象。然后,可以通过调用GeoJsonFeature类中的方法来获取要素的类型和坐标。
相关推荐
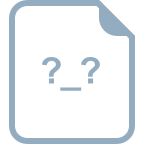
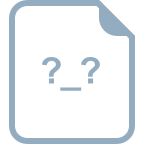














