components组件用法
时间: 2023-10-31 17:04:50 浏览: 130
Components 组件是 Vue.js 框架中的一个重要概念,用于构建可重用的界面元素。通过组合多个组件,可以轻松地构建复杂的用户界面。在 Vue.js 中,每个组件都拥有各自独立的作用域,这意味着它们可以包含自己的模板、样式和行为逻辑。
要使用 Components 组件,首先需要在 Vue.js 代码中定义组件,然后在模板中将其作为标签引入。组件中可以包含多个子组件,并且可以通过插槽(slot)机制动态地插入内容。
在使用 Components 组件时,需要注意组件的命名规范、数据绑定和事件监听等方面的问题。Vue.js 的文档中提供了详细的使用说明和示例代码。
相关问题
vue3components注册组件
### 回答1:
Vue3中注册组件的方式有两种:
1. 全局注册组件
在main.js中使用Vue.createApp()创建Vue实例后,可以通过Vue.component()方法全局注册组件,如下所示:
```
import { createApp } from 'vue'
import App from './App.vue'
import HelloWorld from './components/HelloWorld.vue'
const app = createApp(App)
app.component('hello-world', HelloWorld)
app.mount('#app')
```
其中,'hello-world'是组件的名称,HelloWorld是组件的定义。
2. 局部注册组件
在组件内部使用components选项局部注册组件,如下所示:
```
<template>
<div>
<hello-world></hello-world>
</div>
</template>
<script>
import HelloWorld from './HelloWorld.vue'
export default {
name: 'MyComponent',
components: {
'hello-world': HelloWorld
}
}
</script>
```
其中,'hello-world'是组件的名称,HelloWorld是组件的定义。在模板中使用组件时,需要使用组件的名称来引用。
### 回答2:
Vue 3是目前Vue.js框架的最新版本,与Vue 2相比, Vue 3的性能和体验均有所提升。在Vue 3中,组件注册的方式也有了一些变化。
1.全局注册:
Vue 3中,我们可以使用Vue.createApp方法来创建一个新的Vue实例,然后使用全局API来注册组件。例如:
```
const app = Vue.createApp({});
app.component('hello-world', {
template: '<h1>Hello World!</h1>'
})
app.mount('#app');
```
在这个例子中,我们首先使用createApp方法创建了一个新的Vue 3实例,并且使用component方法来注册了一个名称为hello-world的组件。这个组件中的template属性指定了该组件的渲染模板。
然后,我们使用mount方法将这个app实例挂载到页面中的id为app的DOM节点上。当Vue实例挂载到DOM节点时,hello-world组件将自动呈现在页面上。
2.局部注册:
除了全局注册外,Vue 3也支持将组件作为一个对象传入另一个组件的components选项中进行局部注册。例如:
```
const HelloWorld = {
template: '<h1>Hello World!</h1>'
}
const App = {
components: {
'hello-world': HelloWorld
},
template: '<div><hello-world></hello-world></div>'
}
Vue.createApp(App).mount('#app');
```
在这个例子中,我们首先定义了一个名为HelloWorld的组件对象。然后,我们在App组件的components选项中将这个组件作为局部组件进行了注册。最后,我们将App组件实例化并将其挂载到DOM节点上。
注意,局部注册的组件只能在它们所在的组件中使用,而全局注册的组件可以在整个应用程序中使用。
3.懒加载注册:
当我们的应用变得越来越复杂时,引入的组件数量也会变多,这可能会导致应用程序加载缓慢。这时,Vue 3可以使用懒加载的方式来注册组件,只有在需要使用该组件时,才会进行实际的加载。
例如:
```
const HelloWorld = () => import('./HelloWorld.vue')
const app = Vue.createApp({});
app.component('hello-world', HelloWorld)
app.mount('#app')
```
在这个例子中,我们使用了ES6的箭头函数来定义一个动态导入的组件HelloWorld,并在全局注册时将其作为参数传入component方法中进行组件的注册。这样,当我们需要使用HelloWorld组件时,Vue 3才会从服务器上下载并加载它,以达到最大程度的性能优化。
总结:
以上就是Vue 3中组件注册的方式。在Vue 3中,我们可以使用全局注册、局部注册和懒加载注册等不同的方式来注册组件,以适应不同的应用场景和业务需求。无论何种方式,Vue 3都可以帮助我们更高效地创建组件,并提供更好的性能和用户体验。
### 回答3:
Vue.js 是一款非常流行的前端框架,它提供了丰富的组件化开发方式,可以让我们轻松地组织代码、分离关注点、复用代码等。Vue 3 作为 Vue.js 的最新版本,为我们带来了更强大的组件化开发能力。
Vue3 组件注册包括全局组件注册和局部组件注册两种方式,下面我们分别来看一下。
全局组件注册
全局组件可以在任何组件中使用,我们可以通过 Vue.component() 函数来进行全局组件的注册。
Vue.component('my-component', {
template: '<div>这是一个全局组件</div>'
})
然后在任何组件中即可直接使用, 如:
<template>
<div>
<my-component></my-component>
</div>
</template>
局部组件注册
局部组件只能在其父组件及其子组件中使用,一般情况下我们优先使用局部组件,可以提高代码的复用性和可维护性。
局部组件注册有两种方式,分别是:
1. 对象形式
在父组件中通过 components 配置项来注册局部组件,如:
<template>
<div>
<my-component></my-component>
</div>
</template>
<script>
import MyComponent from './MyComponent.vue';
export default {
components: {
'my-component': MyComponent
}
}
</script>
2. 函数形式
如果我们希望注册的组件只在父级组件中可用,可以将组件注册成一个函数。函数的返回值就是我们要注册的组件。
在父组件中通过 components 配置项进行注册,如:
<template>
<div>
<my-component></my-component>
</div>
</template>
<script>
export default {
components: {
'my-component': () => import('./MyComponent.vue')
}
}
</script>
到此,Vue3 组件注册就讲解完了。总体来说,Vue3 对组件化开发做了很大的优化,让我们能更加便捷地进行组件化开发,提高代码的可读性和可维护性。
vue3 components 引用组件
在Vue3中,引用组件的方式与Vue2有所不同。Vue3使用了新的组件注册方法createApp(),可以通过以下步骤引用组件:
1. 在组件文件中,使用export default导出组件对象。
2. 在父组件中,使用import语句引入子组件。
3. 在父组件中,使用createApp()方法创建Vue实例,并在components选项中注册子组件。
4. 在父组件的template中使用子组件的标签名即可引用子组件。
示例代码如下:
// 子组件文件 ChildComponent.vue
<template>
<div>我是子组件</div>
</template>
<script>
export default {
name: 'ChildComponent'
}
</script>
// 父组件文件 ParentComponent.vue
<template>
<div>
<h1>我是父组件</h1>
<ChildComponent />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue'
import { createApp } from 'vue'
export default {
name: 'ParentComponent',
components: {
ChildComponent
}
}
</script>
// 在入口文件 main.js 中创建Vue实例
import ParentComponent from './ParentComponent.vue'
import { createApp } from 'vue'
createApp(ParentComponent).mount('#app')
在上述代码中,我们首先在子组件文件中导出了组件对象,并在父组件文件中使用import语句引入了子组件。然后,在父组件文件中使用createApp()方法创建Vue实例,并在components选项中注册了子组件。最后,在父组件的template中使用子组件的标签名ChildComponent即可引用子组件。在入口文件main.js中,我们创建了Vue实例并将其挂载到了页面上。
需要注意的是,在Vue3中,组件名不再需要使用kebab-case命名法,可以使用camelCase或PascalCase命名法。同时,组件的template选项也被废弃了,可以使用单文件组件的方式编写组件模板。
阅读全文
相关推荐
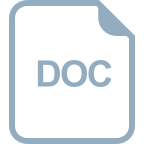
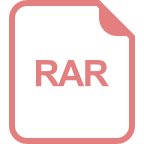
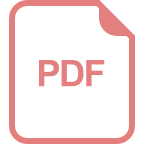
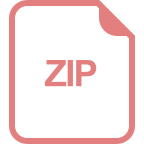
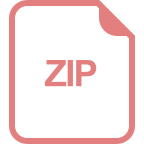
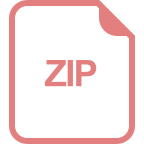
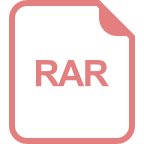
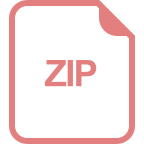
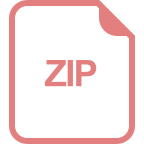
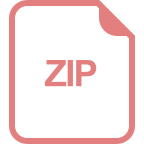
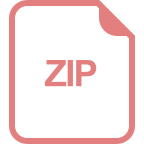
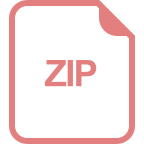
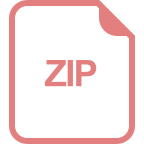
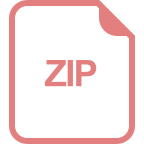
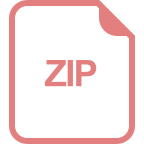
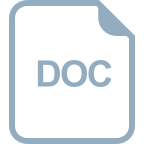