#include <stdio.h> #include <string.h> int compress(char s[]) { int i, j, count = 0; for (i = 0, j = 0; s[i] != '\0'; i++) { if (s[i] == s[j]) { count++; } else { if (count > 1) { s[++j] = count + '0'; } s[++j] = s[i]; count = 1; } } if (count > 1) { s[++j] = count + '0'; } s[++j] = '\0'; return i - j; } int main() { char s[100]; fgets(s, sizeof(s), stdin); int deleted_chars = compress(s); printf("%s\n%d", s, deleted_chars); return 0; }请对其纠错
时间: 2023-11-28 22:47:09 浏览: 49
纠错如下:
1. 在第一行和第二行之间加一个空格。
2. 在 `compress` 函数的开头,将 `count` 初始化为 1,因为第一个字符始终存在于压缩后的字符串中。
3. 在判断 `count` 大于 1 时,需要将 `j` 向前移动一位,因为要将数字插入到当前字符的前面。
4. 在最后返回 `i - j - 1`,因为最后一个字符不需要删除。
修改后的代码如下:
```c
#include <stdio.h>
#include <string.h>
int compress(char s[]) {
int i, j, count = 1;
for (i = 1, j = 0; s[i] != '\0'; i++) {
if (s[i] == s[j]) {
count++;
} else {
if (count > 1) {
j++;
s[j] = count + '0';
}
j++;
s[j] = s[i];
count = 1;
}
}
if (count > 1) {
j++;
s[j] = count + '0';
}
j++;
s[j] = '\0';
return i - j - 1;
}
int main() {
char s[100];
fgets(s, sizeof(s), stdin);
int deleted_chars = compress(s);
printf("%s\n%d", s, deleted_chars);
return 0;
}
```
阅读全文
相关推荐

















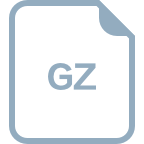
