在数据库中创建商品表goods,表结构如下: number(文本),name(文本),madeTime(日期),price(数字,双精度) 其中number为主键,表示商品编号。 往数据表随机写入30-50条数据。 编写JAVA程序,先查询出goods表中的数据,然后随机获取其中的10条记录,并计算出这10条记录price字段值的平均值,即平均价格,在控制台打印出10条记录和平均价格
时间: 2024-04-10 14:27:30 浏览: 34
你可以使用以下的Java程序来实现查询goods表并计算平均价格的功能:
```java
import java.sql.*;
public class CalculateAveragePrice {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/shop";
String username = "your-username";
String password = "your-password";
try {
// 连接数据库
Connection connection = DriverManager.getConnection(url, username, password);
// 创建查询语句
String query = "SELECT * FROM goods";
// 执行查询
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(query);
// 随机获取10条记录并计算平均价格
double total = 0;
int count = 0;
while (resultSet.next()) {
String number = resultSet.getString("number");
String name = resultSet.getString("name");
Date madeTime = resultSet.getDate("madeTime");
double price = resultSet.getDouble("price");
// 打印每条记录
System.out.println("Number: " + number);
System.out.println("Name: " + name);
System.out.println("Made Time: " + madeTime);
System.out.println("Price: " + price);
System.out.println();
// 计算总价格
total += price;
count++;
// 随机获取10条记录
if (count >= 10) {
break;
}
}
// 计算平均价格
double averagePrice = total / count;
// 打印平均价格
System.out.println("Average Price: " + averagePrice);
// 关闭连接
resultSet.close();
statement.close();
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在上述代码中,你需要将`url`、`username`、`password`替换为你的MySQL数据库连接信息。程序连接到数据库后,执行查询语句`SELECT * FROM goods`获取所有goods表的记录。然后,随机获取10条记录,并计算这10条记录的price字段值的平均值。最后,将每条记录和平均价格打印在控制台上。
你可以运行以上的Java程序来实现你的需求。记得将MySQL数据库驱动添加到你的项目中。
相关推荐
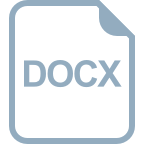
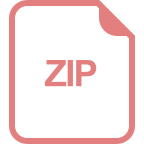
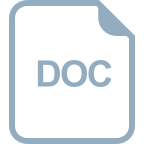












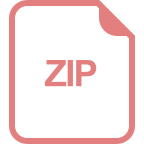
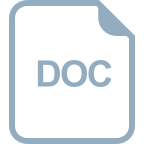
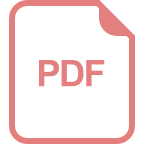
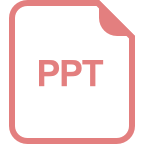