使用C++简单写一个基于面向对象思想,分二级建立王者荣耀游戏中的英雄类(抽象的英雄、特定类型的英雄)。在类中对英雄的属性(比如英雄的种类、魔法值、生命值、物理攻击值)及行为(比如魔法攻击、物理攻击)进行具体描述。随机建立两个英雄,设计PK规则,实现两个英雄的PK,直至分开胜负,并且在PK过程中输出两个英雄的状态变化。的代码
时间: 2024-04-03 11:32:33 浏览: 138
以下是基于面向对象思想的C++代码,实现了英雄类的基本属性和行为,并且设计了简单的PK规则,实现了两个英雄的PK过程。
```c++
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
// 随机函数,用于产生随机数
int random(int min, int max) {
return rand() % (max - min + 1) + min;
}
// 英雄类,作为抽象类,包含公共属性和行为
class Hero {
public:
string name; // 英雄名称
int type; // 英雄类型
int hp; // 生命值
int mp; // 魔法值
int atk; // 物理攻击值
// 构造函数,用于初始化英雄属性
Hero(string name, int type, int hp, int mp, int atk): name(name), type(type), hp(hp), mp(mp), atk(atk) {}
// 英雄的物理攻击行为
virtual void attack(Hero& other) {
int damage = random(atk/2, atk);
cout << name << "对" << other.name << "造成了" << damage << "点伤害" << endl;
other.hp -= damage;
if (other.hp <= 0) {
other.hp = 0;
cout << other.name << "已死亡" << endl;
}
}
// 英雄的魔法攻击行为,由于不是所有英雄都能施展魔法攻击,因此此处为虚函数,需要子类进行重写
virtual void magic(Hero& other) {}
// 英雄的状态输出
void status() {
cout << name << " 状态:" << endl;
cout << "生命值:" << hp << endl;
cout << "魔法值:" << mp << endl;
}
};
// 特定类型英雄类,继承自英雄类,重写了魔法攻击行为
class SpecificHero: public Hero {
public:
SpecificHero(string name, int type, int hp, int mp, int atk): Hero(name, type, hp, mp, atk) {}
// 特定类型英雄的魔法攻击行为
void magic(Hero& other) {
if (mp < 10) {
cout << name << " 没有足够的魔法值施展魔法攻击" << endl;
return;
}
int damage = random(atk, atk*2);
cout << name << "对" << other.name << "施展了魔法攻击,造成了" << damage << "点伤害" << endl;
other.hp -= damage;
mp -= 10;
if (other.hp <= 0) {
other.hp = 0;
cout << other.name << "已死亡" << endl;
}
}
};
int main() {
// 初始化随机数种子
srand(time(NULL));
// 创建两个英雄
Hero* hero1 = new SpecificHero("李白", 1, 100, 50, 20);
Hero* hero2 = new Hero("关羽", 2, 200, 0, 30);
// 输出英雄状态
hero1->status();
hero2->status();
// 开始PK
while (hero1->hp > 0 && hero2->hp > 0) {
int attackOrder = random(1, 2); // 随机攻击顺序
if (attackOrder == 1) {
hero1->attack(*hero2);
if (hero2->hp > 0) {
hero2->attack(*hero1);
}
} else {
hero2->attack(*hero1);
if (hero1->hp > 0) {
hero1->attack(*hero2);
}
}
// 输出状态变化
hero1->status();
hero2->status();
cout << endl;
}
// 输出胜负结果
if (hero1->hp > 0) {
cout << hero1->name << "获得胜利!" << endl;
} else {
cout << hero2->name << "获得胜利!" << endl;
}
// 释放内存
delete hero1;
delete hero2;
return 0;
}
```
在程序中,我们创建了两个英雄,一个是特定类型的英雄“李白”,另一个是抽象的英雄“关羽”。这两个英雄都有自己的属性和行为,其中特定类型的英雄还重写了魔法攻击行为。
在PK过程中,我们采用了随机攻击顺序的方式,每轮攻击后都会输出英雄的状态变化,直至分出胜负。最后我们释放了动态分配的内存。
阅读全文
相关推荐
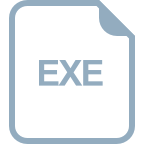
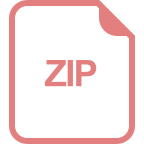
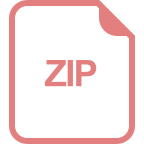
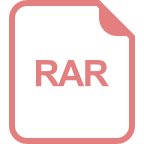
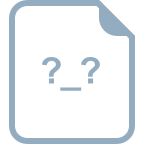
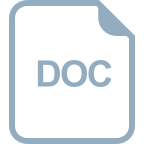
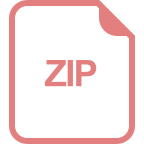
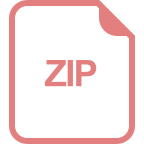
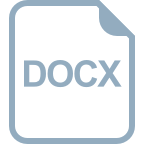
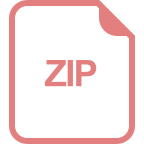
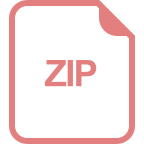
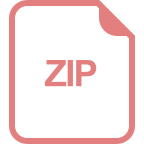
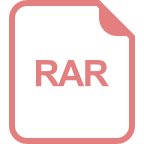
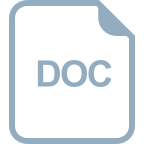