Python中如何分别用于将小写字母转换为大写和将大写字母转换为小写
时间: 2024-02-05 14:12:52 浏览: 79
在Python中,可以使用字符串对象的`upper()`和`lower()`方法将字符串转换为大写或小写。
- `upper()`方法将字符串中的所有小写字母转换为大写字母,并返回转换后的字符串。
- `lower()`方法将字符串中的所有大写字母转换为小写字母,并返回转换后的字符串。
以下是示例代码:
```python
# 将小写字母转换为大写字母
string1 = 'hello world'
string1_upper = string1.upper()
print(string1_upper) # 输出: HELLO WORLD
# 将大写字母转换为小写字母
string2 = 'HELLO WORLD'
string2_lower = string2.lower()
print(string2_lower) # 输出: hello world
```
需要注意的是,`upper()`和`lower()`方法只能处理英文字符,对于其他字符(如中文字符)不起作用。如果需要转换其他字符集,需要使用相应的编码转换函数。
相关问题
定义两个函数,分别用于将小写字母转换为大写和将大写字母转换为小写
以下是 Python 语言的代码实现:
```python
def to_uppercase(s):
"""
将字符串 s 中的小写字母转换为大写字母
"""
return s.upper()
def to_lowercase(s):
"""
将字符串 s 中的大写字母转换为小写字母
"""
return s.lower()
```
使用示例:
```python
>>> s = "Hello World!"
>>> to_uppercase(s)
'HELLO WORLD!'
>>> to_lowercase(s)
'hello world!'
```
定义两个函数,分别用于将小写字母转换为大写和将大写字母转换成小写
下面是两个Python函数,分别用于将小写字母转换为大写和将大写字母转换成小写:
```python
def to_upper(s):
"""
将字符串中的小写字母转换为大写字母
"""
result = ''
for c in s:
if 'a' <= c <= 'z':
result += chr(ord(c) - 32)
else:
result += c
return result
def to_lower(s):
"""
将字符串中的大写字母转换为小写字母
"""
result = ''
for c in s:
if 'A' <= c <= 'Z':
result += chr(ord(c) + 32)
else:
result += c
return result
```
这两个函数都使用了ASCII码来实现字母的大小写转换。具体地,小写字母的ASCII码范围是97~122,而大写字母的ASCII码范围是65~90。因此,在`to_upper`函数中,我们将小写字母的ASCII码减去32,就可以得到对应的大写字母的ASCII码;在`to_lower`函数中,我们将大写字母的ASCII码加上32,就可以得到对应的小写字母的ASCII码。然后,我们再通过`chr`函数将ASCII码转换为对应的字符。
阅读全文
相关推荐
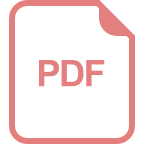
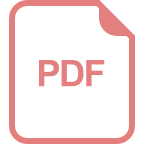














