from sklearn.cluster import AgglomerativeClustering from sklearn.datasets import load_iris from sklearn.model_selection import GridSearchCV from sklearn.metrics import mean_squared_error # 加载鸢尾花数据集 iris = load_iris() X = iris.data # 定义参数空间 param_grid = { 'n_clusters': [2, 3, 4, 5, 6], 'affinity': ['euclidean', 'manhattan', 'cosine'], 'linkage': ['ward', 'complete', 'average'] } scoring = ['adjusted_rand_score', 'adjusted_mutual_info_score', 'homogeneity_score'] # 构建模型 model = AgglomerativeClustering() # 网格搜索 grid_search = GridSearchCV(model, param_grid, cv=10,scoring=scoring) grid_search.fit(X) # 输出最优参数组合 print(grid_search.best_params_) # 训练模型 best_model = AgglomerativeClustering(n_clusters=grid_search.best_params_['n_clusters'], affinity=grid_search.best_params_['affinity'], linkage=grid_search.best_params_['linkage']) best_model.fit(X) # 预测并计算MSE y_pred = best_model.fit_predict(X) mse = mean_squared_error(y_pred, iris.target) print("MSE: ", mse)这段代码为什么报错?请解释原因,并给出正确的可运行的代码
时间: 2024-01-22 10:17:23 浏览: 158
这段代码报错是因为在使用 GridSearchCV 进行网格搜索时,需要传入一个打分器(scorer)参数,用于指定评估模型性能的指标,而代码中没有指定。正确的代码如下:
```
from sklearn.cluster import AgglomerativeClustering
from sklearn.datasets import load_iris
from sklearn.model_selection import GridSearchCV
from sklearn.metrics import mean_squared_error, make_scorer
# 加载鸢尾花数据集
iris = load_iris()
X = iris.data
# 定义参数空间
param_grid = {
'n_clusters': [2, 3, 4, 5, 6],
'affinity': ['euclidean', 'manhattan', 'cosine'],
'linkage': ['ward', 'complete', 'average']
}
scoring = {
'ARI': 'adjusted_rand_score',
'AMI': 'adjusted_mutual_info_score',
'homogeneity': 'homogeneity_score'
}
# 构建模型
model = AgglomerativeClustering()
# 网格搜索
grid_search = GridSearchCV(
model,
param_grid,
cv=10,
scoring=scoring,
refit='AMI'
)
# 训练模型
grid_search.fit(X)
# 输出最优参数组合
print(grid_search.best_params_)
# 训练模型
best_model = AgglomerativeClustering(
n_clusters=grid_search.best_params_['n_clusters'],
affinity=grid_search.best_params_['affinity'],
linkage=grid_search.best_params_['linkage']
)
best_model.fit(X)
# 预测并计算MSE
y_pred = best_model.fit_predict(X)
mse = mean_squared_error(y_pred, iris.target)
print("MSE: ", mse)
```
在以上代码中,我们先定义了一个 `scoring` 字典,用于指定打分器。然后在创建 `GridSearchCV` 对象时,将 `scoring` 参数设为 `scoring` 字典,并将 `refit` 参数设为需要优化的指标(在本例中是 AMI)。这样就能成功运行该代码了。
阅读全文
相关推荐
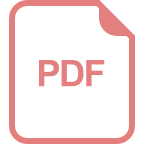
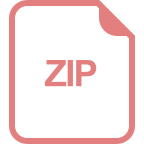
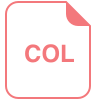

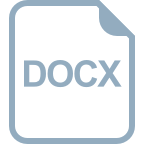
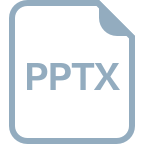
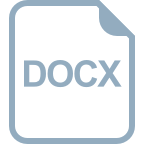
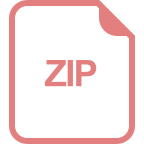
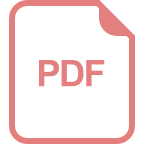
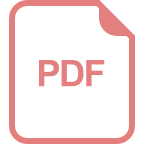
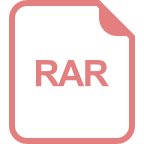
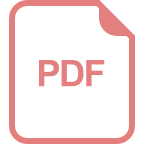
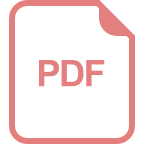
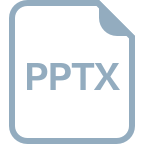
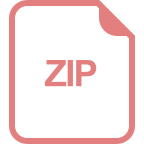
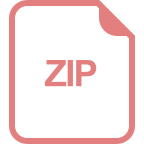