please using cplusplus amake a accumulator,add function add subtract, multiply and divide and make a demo
时间: 2024-05-12 11:17:16 浏览: 22
Sure, I can help you with that! Here's a C++ code for accumulator, with functions to add, subtract, multiply, and divide:
```cpp
#include <iostream>
using namespace std;
class Accumulator {
private:
int value;
public:
Accumulator(int v = 0) : value(v) {}
int getValue() {
return value;
}
void add(int n) {
value += n;
}
void subtract(int n) {
value -= n;
}
void multiply(int n) {
value *= n;
}
void divide(int n) {
if (n == 0) {
cout << "Error: Division by zero!" << endl;
return;
}
value /= n;
}
};
int main() {
Accumulator acc(10);
cout << "Value: " << acc.getValue() << endl;
acc.add(5);
cout << "After adding 5: " << acc.getValue() << endl;
acc.subtract(2);
cout << "After subtracting 2: " << acc.getValue() << endl;
acc.multiply(3);
cout << "After multiplying by 3: " << acc.getValue() << endl;
acc.divide(0);
acc.divide(2);
cout << "After dividing by 2: " << acc.getValue() << endl;
return 0;
}
```
This will create an accumulator object `acc` with an initial value of 10. The `getValue()` function returns the current value of the accumulator. The `add()`, `subtract()`, `multiply()`, and `divide()` functions modify the accumulator value accordingly.
In the `main()` function, we demonstrate how to use these functions by adding 5, subtracting 2, multiplying by 3, and dividing by 0 (which will show an error) and 2.
Hope this helps!
相关推荐
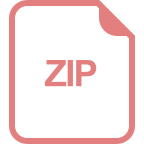
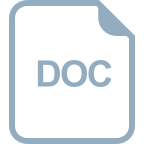














