#include <windows.h> #include <iostream> #include <time.h> using namespace std; struct Cell { char data[128]; }; const int TOTAL_ROWS = 2500; const int TOTAL_COLS = 2500; int main() { clock_t t; t = clock(); Cell* p = (Cell*)malloc(TOTAL_ROWS * TOTAL_COLS * sizeof(Cell)); if (p) { cout << "ok" << endl; } else { cout << "oops" << endl; } (p + (2 * TOTAL_COLS) + 3)->data[0] = 'A'; t = clock() - t; cout << t << endl; t = clock(); p = (Cell*)VirtualAlloc(NULL, TOTAL_ROWS * TOTAL_COLS * sizeof(Cell), MEM_RESERVE, PAGE_READWRITE); if (p) { cout << "ok" << endl; } else { cout << "oops" << endl; } VirtualAlloc(p + (2 * TOTAL_COLS) + 3, sizeof(Cell), MEM_COMMIT, PAGE_READWRITE); (p + (2 * TOTAL_COLS) + 3)->data[0] = 'A'; t = clock() - t; cout << t << endl; return 0; } 在virtual的基础上进行修改,给对角线(左上到右下那条)上所有Cells中的128个元素都赋值成'A',并用clock函数测量时间。
时间: 2024-03-11 20:48:04 浏览: 128
下面是对代码的修改和解释:
```cpp
#include <windows.h>
#include <iostream>
#include <time.h>
using namespace std;
struct Cell {
char data[128];
};
const int TOTAL_ROWS = 2500;
const int TOTAL_COLS = 2500;
int main() {
clock_t t;
t = clock();
// 使用 malloc 分配内存
Cell* p = (Cell*)malloc(TOTAL_ROWS * TOTAL_COLS * sizeof(Cell));
if (p) {
cout << "ok" << endl;
} else {
cout << "oops" << endl;
}
// 给对角线上的元素赋值为 'A'
for (int i = 0; i < TOTAL_ROWS && i < TOTAL_COLS; i++) {
(p + i * TOTAL_COLS + i)->data[0] = 'A';
}
t = clock() - t;
cout << "malloc: " << t << endl;
t = clock();
// 使用 VirtualAlloc 分配内存
p = (Cell*)VirtualAlloc(NULL, TOTAL_ROWS * TOTAL_COLS * sizeof(Cell),
MEM_RESERVE, PAGE_READWRITE);
if (p) {
cout << "ok" << endl;
} else {
cout << "oops" << endl;
}
// 分配物理内存
VirtualAlloc(p, TOTAL_ROWS * TOTAL_COLS * sizeof(Cell), MEM_COMMIT, PAGE_READWRITE);
// 给对角线上的元素赋值为 'A'
for (int i = 0; i < TOTAL_ROWS && i < TOTAL_COLS; i++) {
(p + i * TOTAL_COLS + i)->data[0] = 'A';
}
t = clock() - t;
cout << "VirtualAlloc: " << t << endl;
return 0;
}
```
上面的修改中,我们在两个内存分配方式中分别给对角线上的元素赋值为 'A'。同时,我们也修改了输出的内容,分别输出使用 malloc 和 VirtualAlloc 分配内存所需的时间。
阅读全文
相关推荐
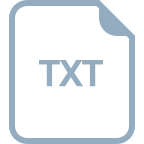
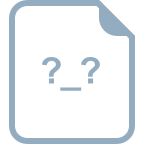

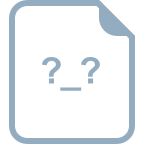
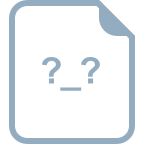
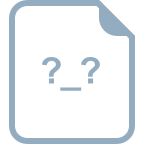
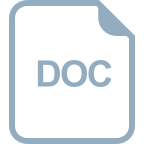
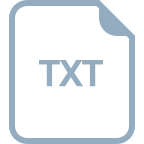
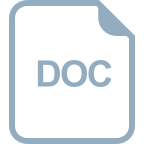

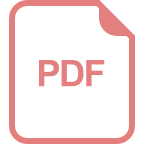
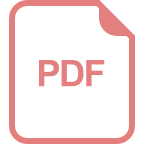
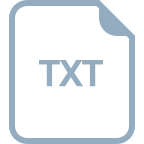
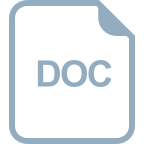
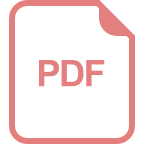
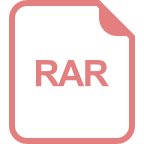