ftpfile如何转成字节流
时间: 2024-03-17 19:44:27 浏览: 18
可以使用Java中的InputStream读取FTP文件并将其转换为字节数组,代码示例如下:
```java
public byte[] ftpFileToBytes(FTPClient ftpClient, String filePath) throws IOException {
InputStream inputStream = ftpClient.retrieveFileStream(filePath);
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
byteArrayOutputStream.write(buffer, 0, bytesRead);
}
ftpClient.completePendingCommand();
inputStream.close();
byteArrayOutputStream.close();
return byteArrayOutputStream.toByteArray();
}
```
其中ftpClient是FTP客户端连接对象,filePath是FTP文件路径。该方法返回FTP文件的字节数组。
相关问题
java将FTPFile转为MultipartFile
根据提供的引用内容,我们发现是通过将`File`对象封装成`MultipartFile`对象来实现转换的。但是`FTPFile`并不是与`File`对象兼容的类型,因此我们需要先将`FTPFile`对象转换为`File`对象,然后再进行封装成`MultipartFile`对象。下面是一个实现该功能的示例代码:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.springframework.mock.web.MockMultipartFile;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
public class FTPFileToMultipartFile {
public static MultipartFile convert(FTPClient ftpClient, String remoteFilePath) throws IOException {
InputStream inputStream = null;
ByteArrayOutputStream outputStream = null;
MultipartFile multipartFile = null;
try {
inputStream = ftpClient.retrieveFileStream(remoteFilePath);
// 将FTP文件流转换为字节数组输出流
outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, len);
}
// 将字节数组输出流转换为字节数组
byte[] fileBytes = outputStream.toByteArray();
// 将字节数组转换为MultipartFile对象
multipartFile = new MockMultipartFile(remoteFilePath,
remoteFilePath.substring(remoteFilePath.lastIndexOf("/") + 1),
"application/octet-stream", new ByteArrayInputStream(fileBytes));
} catch (IOException e) {
e.printStackTrace();
throw new IOException("Convert failed!");
} finally {
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (outputStream != null) {
try {
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (ftpClient != null && inputStream != null && !ftpClient.completePendingCommand()) {
ftpClient.logout();
ftpClient.disconnect();
}
}
return multipartFile;
}
}
```
这个示例代码将FTPFile对象转换为MultipartFile对象,首先获取FTPFile的流,并将其读取到字节数组中,然后通过`MockMultipartFile`对象封装成MultipartFile对象。要使用此方法,需要在类路径中添加`commons-net`和`spring-test`两个jar包。因为我们使用了`FTPClient`来读取远程文件流,同时也使用了`MockMultipartFile`来封装成MultipartFile对象。
kafka可以传输file
Kafka本身并不支持文件传输,但是可以通过将文件内容转换为字节流的方式传输文件。可以将文件读入内存,然后将其转换为字节流,发送到Kafka的Topic中。消费者可以从该Topic中读取字节流并将其转换回文件。这种方式不太适合大文件传输,因为会占用较多的内存和网络带宽。对于大文件传输,可以考虑使用专门的文件传输协议,如FTP或者HTTP等。
相关推荐
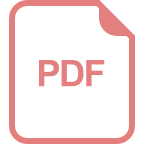
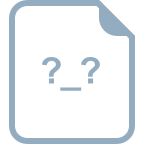
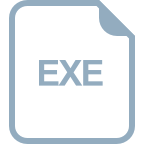











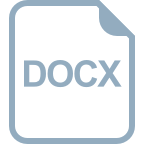
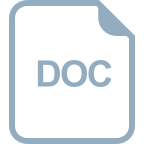