class Person{ public Person(){ System.out.println(“this is a Person”); } } public class Teacher extends Person{ private String name=”tom”; public Teacher(){ System.out.println(“this is a teacher”); super(); } public static void main(String[] args){ Teacher teacher = new Teacher(); System.out.println(this.name); } }
时间: 2024-03-25 21:39:29 浏览: 24
这段代码存在两个问题:
1. `System.out.println(this.name);` 这行代码会导致编译错误,因为 `this` 关键字只能在实例方法中使用,而 `main` 方法是一个静态方法,不能使用 `this` 关键字。
2. 在 `Teacher` 类的构造方法中,调用了 `super()` 方法,表示调用父类 `Person` 的无参构造方法。但是 `Person` 类并没有定义无参构造方法,只有一个无参的默认构造方法。因此,编译器会自动生成一个默认的无参构造方法。但是,如果 `Person` 类中定义了有参构造方法,那么在 `Teacher` 类的构造方法中就必须显式地调用父类的有参构造方法,否则会编译错误。
下面是修改后的代码:
```java
class Person {
public Person(String type){
System.out.println(type + " is a Person");
}
}
public class Teacher extends Person {
private String name = "tom";
public Teacher(){
super("Teacher");
System.out.println("this is a teacher");
}
public static void main(String[] args){
Teacher teacher = new Teacher();
System.out.println(teacher.name);
}
}
```
运行结果:
```
Teacher is a Person
this is a teacher
tom
```
相关推荐
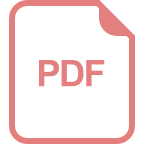
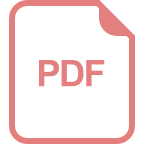
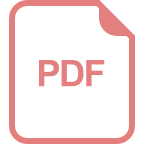















