class Mlp(nn.Module): """ MLP as used in Vision Transformer, MLP-Mixer and related networks """ def __init__( self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, norm_layer=nn.BatchNorm2d, bias=True, drop=0.1, use_conv=False, expan_ratio=6 ): super().__init__() out_features = out_features or in_features hidden_features = hidden_features or in_features bias = to_2tuple(bias) drop_probs = to_2tuple(drop) linear_layer = partial(nn.Conv2d, kernel_size=1) if use_conv else nn.Linear #self.fc1 = linear_layer(in_features, hidden_features, bias=bias[0]) self.pwconv1 = linear_layer(in_features, expan_ratio * hidden_features,bias=bias[0]) self.act = act_layer() self.drop1 = nn.Dropout(drop_probs[0]) self.norm = norm_layer(hidden_features) if norm_layer is not None else nn.Identity() #self.fc2 = linear_layer(hidden_features, out_features, bias=bias[1]) self.pwconv2 = linear_layer(expan_ratio * hidden_features,out_features,bias=bias[1]) self.drop2 = nn.Dropout(drop_probs[1]) def forward(self, x): B, N, C = x.shape #x = self.fc1(x) x = self.pwconv1(x) x = self.act(x) x = self.drop1(x) #x = self.fc2(x) x = self.pwconv2(x) x = self.drop2(x) return x 哪里有问题
时间: 2023-06-18 20:05:42 浏览: 163
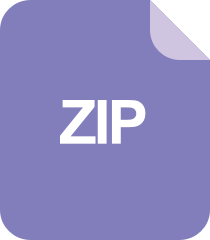
BPNN-CPP.zip_BPNN-GA_GA-MLP NN
这是一个MLP类的定义,其中使用了partial函数来定义一个带有默认参数的函数。在__init__函数中,使用linear_layer函数创建了两个线性层(或者卷积层,取决于use_conv参数),分别是pwconv1和pwconv2。其中pwconv1将输入特征in_features通过一个扩张系数expan_ratio扩张,输出hidden_features。pwconv2将输入特征expan_ratio * hidden_features通过一个扩张系数1,输出out_features。这个类的forward函数将输入x通过pwconv1、激活函数、dropout、pwconv2、dropout的顺序进行计算,最终输出x。根据注释掉的代码,这里使用了卷积层或线性层来实现MLP。至于哪里有问题,就需要看具体的使用情况和期望的结果。
阅读全文
相关推荐
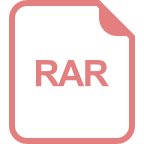
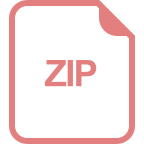

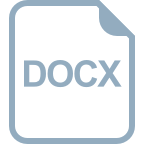
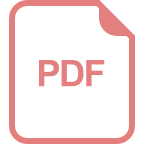
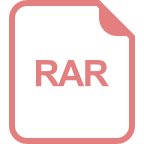
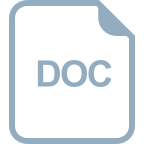
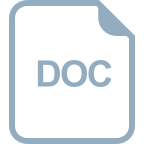
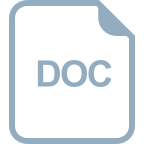
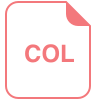






