Android 7.0.2自定义Toast显示时间 通过longclick触发,显示200秒,setDuration不好使
时间: 2023-12-03 07:42:37 浏览: 32
在 Android 中,Toast 类的 `setDuration()` 方法是用来设置 Toast 显示的时间的。但是,它只能接受 Toast.LENGTH_SHORT(短)或 Toast.LENGTH_LONG(长)这两个值。
如果想要自定义 Toast 的显示时间,可以通过以下步骤实现:
1. 创建一个自定义 Toast 的布局文件,在其中添加一个 TextView。
2. 在代码中加载该布局文件,并设置 TextView 的文本。
3. 创建一个 Toast 对象,并将其设置为自定义布局。
4. 调用 Toast 对象的 `setGravity()` 方法设置 Toast 的位置。
5. 调用 Toast 对象的 `show()` 方法显示 Toast。
6. 在长按事件中启动一个定时器,定时隐藏 Toast。
以下是具体的代码实现:
1. 定义一个自定义 Toast 的布局文件(custom_toast.xml):
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp"
android:background="#333333">
<TextView
android:id="@+id/message"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#FFFFFF"
android:textSize="16sp" />
</LinearLayout>
```
2. 在代码中加载该布局文件,并设置 TextView 的文本:
```
LayoutInflater inflater = getLayoutInflater();
View layout = inflater.inflate(R.layout.custom_toast,
(ViewGroup) findViewById(R.id.custom_toast_layout));
TextView text = (TextView) layout.findViewById(R.id.message);
text.setText("自定义 Toast");
```
3. 创建一个 Toast 对象,并将其设置为自定义布局:
```
Toast toast = new Toast(getApplicationContext());
toast.setDuration(Toast.LENGTH_LONG);
toast.setView(layout);
```
4. 调用 Toast 对象的 `setGravity()` 方法设置 Toast 的位置:
```
toast.setGravity(Gravity.CENTER_VERTICAL, 0, 0);
```
5. 调用 Toast 对象的 `show()` 方法显示 Toast:
```
toast.show();
```
6. 在长按事件中启动一个定时器,定时隐藏 Toast:
```
final Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
toast.cancel();
}
}, 200000); // 显示 200 秒后隐藏 Toast
```
完整代码示例:
```
Button button = findViewById(R.id.button);
button.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
LayoutInflater inflater = getLayoutInflater();
View layout = inflater.inflate(R.layout.custom_toast,
(ViewGroup) findViewById(R.id.custom_toast_layout));
TextView text = (TextView) layout.findViewById(R.id.message);
text.setText("自定义 Toast");
Toast toast = new Toast(getApplicationContext());
toast.setDuration(Toast.LENGTH_LONG);
toast.setView(layout);
toast.setGravity(Gravity.CENTER_VERTICAL, 0, 0);
toast.show();
final Handler handler = new Handler();
handler.postDelayed(new Runnable() {
@Override
public void run() {
toast.cancel();
}
}, 200000); // 显示 200 秒后隐藏 Toast
return true;
}
});
```
相关推荐
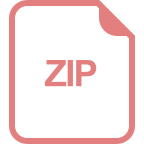
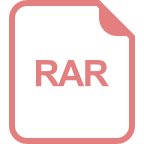
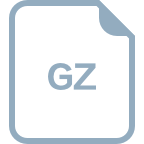













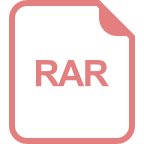
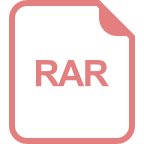