利用jsp,sql实现购物车的相关代码
时间: 2024-02-15 19:05:35 浏览: 94
以下是一个简单的JSP和SQL实现购物车的示例,其中使用了MySQL数据库。
购物车页面(cart.jsp):
```
<%-- 获取购物车中的项目 --%>
<%
String[] itemIds = request.getParameterValues("itemId");
String[] quantities = request.getParameterValues("quantity");
if (itemIds != null && quantities != null) {
for (int i = 0; i < itemIds.length; i++) {
String itemId = itemIds[i];
int quantity = Integer.parseInt(quantities[i]);
// 将项目添加到购物车
addToCart(itemId, quantity);
}
}
// 获取购物车中的所有项目
List<CartItem> cartItems = getCartItems();
%>
<%-- 显示购物车中的项目 --%>
<table>
<thead>
<tr>
<th>名称</th>
<th>价格</th>
<th>数量</th>
</tr>
</thead>
<tbody>
<c:forEach var="cartItem" items="${cartItems}">
<tr>
<td>${cartItem.name}</td>
<td>${cartItem.price}</td>
<td>
<form method="post" action="${pageContext.request.contextPath}/updateCart.jsp">
<input type="hidden" name="itemId" value="${cartItem.itemId}">
<input type="text" name="quantity" value="${cartItem.quantity}">
<input type="submit" value="更新">
</form>
</td>
<td>
<form method="post" action="${pageContext.request.contextPath}/removeFromCart.jsp">
<input type="hidden" name="itemId" value="${cartItem.itemId}">
<input type="submit" value="删除">
</form>
</td>
</tr>
</c:forEach>
</tbody>
</table>
<%-- 显示所有可用项目 --%>
<h2>所有可用项目</h2>
<table>
<thead>
<tr>
<th>名称</th>
<th>价格</th>
<th>数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach var="item" items="${items}">
<tr>
<td>${item.name}</td>
<td>${item.price}</td>
<td>
<form method="post" action="${pageContext.request.contextPath}/cart.jsp">
<input type="hidden" name="itemId" value="${item.itemId}">
<input type="text" name="quantity" value="1">
<input type="submit" value="添加到购物车">
</form>
</td>
</tr>
</c:forEach>
</tbody>
</table>
```
将项目添加到购物车(addToCart.jsp):
```
<%
String itemId = request.getParameter("itemId");
int quantity = Integer.parseInt(request.getParameter("quantity"));
addToCart(itemId, quantity);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
%>
<%-- 将项目添加到购物车 --%>
<%
private void addToCart(String itemId, int quantity) {
// 查询项目的名称和价格
String name = null;
double price = 0.0;
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = getConnection();
stmt = conn.prepareStatement("SELECT name, price FROM items WHERE item_id = ?");
stmt.setString(1, itemId);
rs = stmt.executeQuery();
if (rs.next()) {
name = rs.getString("name");
price = rs.getDouble("price");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
close(rs, stmt, conn);
}
// 如果购物车中已有该项目,则增加数量,否则添加新项目
boolean found = false;
for (CartItem cartItem : cart) {
if (cartItem.getItemId().equals(itemId)) {
cartItem.setQuantity(cartItem.getQuantity() + quantity);
found = true;
break;
}
}
if (!found) {
CartItem cartItem = new CartItem(itemId, name, price, quantity);
cart.add(cartItem);
}
}
%>
```
从购物车中删除项目(removeFromCart.jsp):
```
<%
String itemId = request.getParameter("itemId");
removeFromCart(itemId);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
%>
<%-- 从购物车中删除项目 --%>
<%
private void removeFromCart(String itemId) {
Iterator<CartItem> iterator = cart.iterator();
while (iterator.hasNext()) {
CartItem cartItem = iterator.next();
if (cartItem.getItemId().equals(itemId)) {
iterator.remove();
break;
}
}
}
%>
```
更新购物车中的项目(updateCart.jsp):
```
<%
String itemId = request.getParameter("itemId");
int quantity = Integer.parseInt(request.getParameter("quantity"));
updateCart(itemId, quantity);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
%>
<%-- 更新购物车中的项目 --%>
<%
private void updateCart(String itemId, int quantity) {
for (CartItem cartItem : cart) {
if (cartItem.getItemId().equals(itemId)) {
cartItem.setQuantity(quantity);
break;
}
}
}
%>
```
以上是一个简单的JSP和SQL实现购物车的示例,其中使用了MySQL数据库。实际应用中,可能需要根据具体需求进行修改和优化。
阅读全文
相关推荐
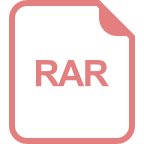

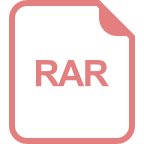
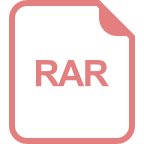
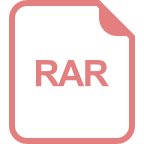
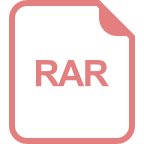





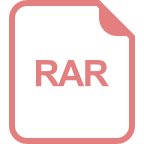
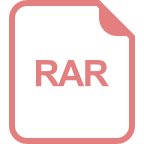

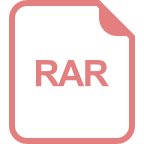
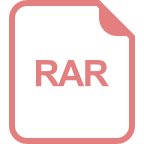