Node.js+MySQL实现的客服系统
时间: 2024-03-31 18:35:21 浏览: 13
以下是一个简单的Node.js和MySQL实现的客服系统的示例代码:
1. 创建数据库和表
首先,需要创建一个MySQL数据库和相应的表,用于存储客户数据和订单信息。可以执行以下SQL语句来创建表:
```
CREATE DATABASE `customer_service`;
USE `customer_service`;
CREATE TABLE `customers` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
`email` varchar(255) NOT NULL,
`phone` varchar(20) NOT NULL,
`message` text NOT NULL,
`created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `orders` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`customer_id` int(11) NOT NULL,
`product` varchar(255) NOT NULL,
`quantity` int(11) NOT NULL,
`price` decimal(10,2) NOT NULL,
`created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`),
KEY `fk_customer_id` (`customer_id`),
CONSTRAINT `fk_customer_id` FOREIGN KEY (`customer_id`) REFERENCES `customers` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
2. 创建Node.js服务端程序
接下来,可以创建一个Node.js服务端程序,用于处理客户端的请求和响应。可以使用Express框架来创建一个简单的HTTP服务,并使用mysql模块来连接MySQL数据库和执行SQL查询。以下是一个示例程序:
```
const express = require('express');
const mysql = require('mysql');
const app = express();
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'customer_service'
});
connection.connect((err) => {
if (err) {
console.error('Error connecting to database: ' + err.stack);
return;
}
console.log('Connected to database with ID: ' + connection.threadId);
});
app.use(express.json());
app.get('/customers', (req, res) => {
connection.query('SELECT * FROM customers', (err, results) => {
if (err) {
console.error('Error executing query: ' + err.stack);
res.status(500).send('Error executing query');
return;
}
res.send(results);
});
});
app.post('/customers', (req, res) => {
const { name, email, phone, message } = req.body;
connection.query('INSERT INTO customers (name, email, phone, message) VALUES (?, ?, ?, ?)', [name, email, phone, message], (err, results) => {
if (err) {
console.error('Error executing query: ' + err.stack);
res.status(500).send('Error executing query');
return;
}
res.send(results);
});
});
app.get('/orders', (req, res) => {
connection.query('SELECT * FROM orders', (err, results) => {
if (err) {
console.error('Error executing query: ' + err.stack);
res.status(500).send('Error executing query');
return;
}
res.send(results);
});
});
app.post('/orders', (req, res) => {
const { customer_id, product, quantity, price } = req.body;
connection.query('INSERT INTO orders (customer_id, product, quantity, price) VALUES (?, ?, ?, ?)', [customer_id, product, quantity, price], (err, results) => {
if (err) {
console.error('Error executing query: ' + err.stack);
res.status(500).send('Error executing query');
return;
}
res.send(results);
});
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
3. 创建Vue客户端程序
最后,可以创建一个Vue客户端程序,用于展示用户界面和发送HTTP请求。可以使用axios模块来发送HTTP请求,并使用Vue组件来创建用户界面。以下是一个示例程序:
```
<template>
<div>
<h2>Customer List</h2>
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
<th>Message</th>
<th>Date</th>
</tr>
</thead>
<tbody>
<tr v-for="customer in customers" :key="customer.id">
<td>{{ customer.name }}</td>
<td>{{ customer.email }}</td>
<td>{{ customer.phone }}</td>
<td>{{ customer.message }}</td>
<td>{{ customer.created_at }}</td>
</tr>
</tbody>
</table>
<h2>Order List</h2>
<table>
<thead>
<tr>
<th>Customer</th>
<th>Product</th>
<th>Quantity</th>
<th>Price</th>
<th>Date</th>
</tr>
</thead>
<tbody>
<tr v-for="order in orders" :key="order.id">
<td>{{ customers.find(c => c.id === order.customer_id).name }}</td>
<td>{{ order.product }}</td>
<td>{{ order.quantity }}</td>
<td>{{ order.price }}</td>
<td>{{ order.created_at }}</td>
</tr>
</tbody>
</table>
<form @submit.prevent="submit">
<h2>Contact Us</h2>
<div>
<label>Name:</label>
<input type="text" v-model="name" required>
</div>
<div>
<label>Email:</label>
<input type="email" v-model="email" required>
</div>
<div>
<label>Phone:</label>
<input type="tel" v-model="phone" required>
</div>
<div>
<label>Message:</label>
<textarea v-model="message" required></textarea>
</div>
<button type="submit">Submit</button>
</form>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
customers: [],
orders: [],
name: '',
email: '',
phone: '',
message: ''
};
},
mounted() {
axios.get('/customers').then(response => {
this.customers = response.data;
});
axios.get('/orders').then(response => {
this.orders = response.data;
});
},
methods: {
submit() {
axios.post('/customers', { name: this.name, email: this.email, phone: this.phone, message: this.message }).then(response => {
alert('Your message has been sent.');
this.name = '';
this.email = '';
this.phone = '';
this.message = '';
});
}
}
};
</script>
```
以上仅是一个简单的示例代码,实际的客服系统可能要更加复杂和完善,需要根据实际业务需求进行设计和开发。
相关推荐
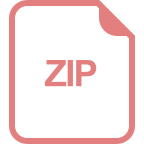
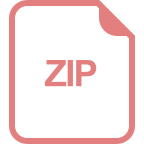
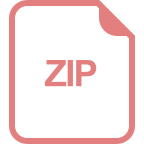














