简单C语言编写函数,其功能分别为: 求N个整数的次大值和次小值并求这两个整数的最大公约数和最小公倍数
时间: 2024-05-11 22:19:04 浏览: 18
以下是一个简单的C语言函数,可以用来实现求N个整数的次大值和次小值,并计算这两个整数的最大公约数和最小公倍数。
```c
#include <stdio.h>
#include <stdlib.h>
int gcd(int a, int b);
int lcm(int a, int b);
int main()
{
int n, temp, max, second_max, min, second_min, i, j;
int gcd_result, lcm_result;
printf("请输入整数个数:");
scanf("%d", &n);
printf("请输入%d个整数:", n);
// 读入第一个数,初始化max、second_max、min、second_min
scanf("%d", &max);
second_max = min = second_min = gcd_result = lcm_result = 0;
scanf("%d", &temp);
// 比较大小,更新max、second_max、min、second_min
if (temp > max) {
second_max = max;
max = temp;
} else {
second_max = temp;
}
min = second_min = gcd_result = lcm_result = temp;
for (i = 2; i < n; i++) {
scanf("%d", &temp);
if (temp > max) {
second_max = max;
max = temp;
} else if (temp > second_max) {
second_max = temp;
}
if (temp < min) {
second_min = min;
min = temp;
} else if (temp < second_min) {
second_min = temp;
}
gcd_result = gcd(gcd_result, temp);
lcm_result = lcm(lcm_result, temp);
}
printf("次大值:%d,次小值:%d,最大公约数:%d,最小公倍数:%d\n", second_max, second_min, gcd_result, lcm_result);
return 0;
}
// 求最大公约数
int gcd(int a, int b)
{
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
// 求最小公倍数
int lcm(int a, int b)
{
return (a * b) / gcd(a, b);
}
```
在该程序中,我们首先要求用户输入整数个数n,然后依次读入n个整数,并在读入每个整数时更新最大值、次大值、最小值和次小值。同时,我们还需要计算这n个整数的最大公约数和最小公倍数。为了实现这一点,我们定义了两个函数gcd和lcm,分别用于计算两个整数的最大公约数和最小公倍数。最后,我们将求出的次大值、次小值、最大公约数和最小公倍数输出到屏幕上。
相关推荐
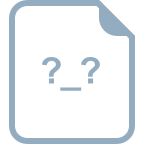
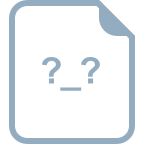














