c 语言输入任意数量单词,每个分别存放
时间: 2024-02-19 10:34:44 浏览: 31
在一个字符串数组中,并输出这些单词。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char words[100][50]; // 定义字符串数组,存放最多100个单词,每个单词最长50个字符
int n = 0; // n表示实际存放的单词数量
// 循环读入单词,直到输入空行为止
while (fgets(words[n], 50, stdin) != NULL && words[n][0] != '\n') {
n++;
}
// 输出所有单词
for (int i = 0; i < n; i++) {
printf("%s", words[i]);
}
return 0;
}
```
在上面的代码中,我们先定义了一个字符串数组`words`,可以存放最多100个单词,每个单词最长50个字符。然后,使用`fgets`函数循环读入单词,直到输入空行为止。最后,使用循环输出所有单词。
注意,`fgets`函数会读入换行符,因此在判断输入是否为空行时,需要判断`words[n][0]`是否为`\n`。
相关问题
c 语言输入已知数量单词,每个分别存放
在一个字符串数组中,然后统计其中包含字母a的单词数量并输出。
可以使用以下代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
char words[10][20]; // 字符串数组,存放单词
int count = 0; // 统计包含字母a的单词数量
// 输入单词
printf("请输入10个单词:\n");
for (int i = 0; i < 10; i++) {
scanf("%s", words[i]);
}
// 统计包含字母a的单词数量
for (int i = 0; i < 10; i++) {
if (strchr(words[i], 'a') != NULL) {
count++;
}
}
// 输出结果
printf("包含字母a的单词数量为:%d\n", count);
return 0;
}
```
其中,`strchr()` 函数用于在字符串中查找指定字符,如果找到则返回该字符在字符串中的指针,否则返回 NULL。在上述代码中,我们使用该函数查找每个单词中是否包含字母a。
c 语言输入已知数量字符串,每个分别存放
在不同的字符数组中,然后将它们按照字典序从小到大排序输出。
可以使用strcmp函数进行字符串比较,然后使用冒泡排序或者快速排序等算法进行排序。具体实现如下:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STR_NUM 100
#define MAX_STR_LEN 100
void bubble_sort(char str[][MAX_STR_LEN], int n)
{
int i, j;
char temp[MAX_STR_LEN];
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (strcmp(str[j], str[j + 1]) > 0) {
strcpy(temp, str[j]);
strcpy(str[j], str[j + 1]);
strcpy(str[j + 1], temp);
}
}
}
}
int main()
{
char strs[MAX_STR_NUM][MAX_STR_LEN];
int n, i;
printf("请输入字符串的数量:");
scanf("%d", &n);
printf("请输入%d个字符串,每个字符串长度不超过%d个字符:\n", n, MAX_STR_LEN - 1);
for (i = 0; i < n; i++) {
scanf("%s", strs[i]);
}
bubble_sort(strs, n);
printf("按照字典序从小到大排序后的字符串为:\n");
for (i = 0; i < n; i++) {
printf("%s\n", strs[i]);
}
return 0;
}
```
这里使用了冒泡排序算法,也可以使用快速排序等其他排序算法。
相关推荐
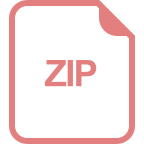












