c语言编程实现:设计3个级别难度的迷宫地图并编程实现电子老鼠走迷宫游戏。 (1)通过键盘交互的方式模拟电子老鼠走出迷宫。 (2)设计程序让电子老鼠自动走出迷宫。 要求: 1)3个级别难度的迷宫地图,规模分别大于12*12、20*20、40*40. 2)迷宫地图保存到文本文件中,程序根据用户选择的难度级别读取文件获取迷宫地图数据。
时间: 2023-11-27 10:50:41 浏览: 160
以下是一个简单的实现,包含了三个难度级别的迷宫地图和一个电子老鼠走迷宫的游戏。这个程序可以在Linux或者Windows平台上编译运行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
#define MAX_LEVEL 3
/* 迷宫地图结构 */
typedef struct {
int width;
int height;
char **data;
} maze_t;
/* 电子老鼠结构 */
typedef struct {
int x;
int y;
} mouse_t;
/* 读取迷宫地图 */
maze_t *load_maze(const char *filename) {
FILE *file = fopen(filename, "r");
if (file == NULL) {
return NULL;
}
maze_t *maze = (maze_t *) malloc(sizeof(maze_t));
if (maze == NULL) {
fclose(file);
return NULL;
}
fscanf(file, "%d %d", &maze->width, &maze->height);
maze->data = (char **) malloc(sizeof(char *) * maze->height);
if (maze->data == NULL) {
free(maze);
fclose(file);
return NULL;
}
for (int y = 0; y < maze->height; ++y) {
maze->data[y] = (char *) malloc(sizeof(char) * (maze->width + 1));
if (maze->data[y] == NULL) {
for (int i = 0; i < y; ++i) {
free(maze->data[i]);
}
free(maze->data);
free(maze);
fclose(file);
return NULL;
}
fscanf(file, "%s", maze->data[y]);
}
fclose(file);
return maze;
}
/* 释放迷宫地图 */
void free_maze(maze_t *maze) {
for (int y = 0; y < maze->height; ++y) {
free(maze->data[y]);
}
free(maze->data);
free(maze);
}
/* 打印迷宫地图 */
void print_maze(const maze_t *maze) {
for (int y = 0; y < maze->height; ++y) {
printf("%s\n", maze->data[y]);
}
}
/* 移动电子老鼠 */
void move_mouse(maze_t *maze, mouse_t *mouse, int dx, int dy) {
int x = mouse->x + dx;
int y = mouse->y + dy;
if (x >= 0 && x < maze->width && y >= 0 && y < maze->height && maze->data[y][x] != '#') {
mouse->x = x;
mouse->y = y;
}
}
/* 通过键盘交互方式模拟电子老鼠走迷宫 */
void play_game_with_keyboard(maze_t *maze, mouse_t *mouse) {
while (1) {
system("cls||clear");
print_maze(maze);
printf("Use arrow keys to move the mouse. Press q to quit.\n");
int c = getchar();
if (c == 'q' || c == 'Q') {
break;
} else if (c == '\033') {
getchar();
c = getchar();
switch (c) {
case 'A': move_mouse(maze, mouse, 0, -1); break;
case 'B': move_mouse(maze, mouse, 0, 1); break;
case 'C': move_mouse(maze, mouse, 1, 0); break;
case 'D': move_mouse(maze, mouse, -1, 0); break;
}
}
}
}
/* 设计程序让电子老鼠自动走出迷宫 */
void play_game_with_ai(maze_t *maze, mouse_t *mouse) {
int dx[4] = {0, 0, 1, -1};
int dy[4] = {-1, 1, 0, 0};
int **visited = (int **) malloc(sizeof(int *) * maze->height);
for (int y = 0; y < maze->height; ++y) {
visited[y] = (int *) malloc(sizeof(int) * maze->width);
for (int x = 0; x < maze->width; ++x) {
visited[y][x] = 0;
}
}
visited[mouse->y][mouse->x] = 1;
while (1) {
int found_exit = 0;
for (int i = 0; i < 4; ++i) {
int x = mouse->x + dx[i];
int y = mouse->y + dy[i];
if (x >= 0 && x < maze->width && y >= 0 && y < maze->height && maze->data[y][x] != '#' && visited[y][x] == 0) {
found_exit = 1;
break;
}
}
if (!found_exit) {
break;
}
int d = rand() % 4;
while (1) {
int x = mouse->x + dx[d];
int y = mouse->y + dy[d];
if (x >= 0 && x < maze->width && y >= 0 && y < maze->height && maze->data[y][x] != '#' && visited[y][x] == 0) {
move_mouse(maze, mouse, dx[d], dy[d]);
visited[mouse->y][mouse->x] = 1;
break;
}
d = rand() % 4;
}
system("cls||clear");
print_maze(maze);
}
for (int y = 0; y < maze->height; ++y) {
free(visited[y]);
}
free(visited);
}
/* 主函数 */
int main() {
const char *filenames[MAX_LEVEL] = {"maze1.txt", "maze2.txt", "maze3.txt"};
int width[MAX_LEVEL] = {12, 20, 40};
int height[MAX_LEVEL] = {12, 20, 40};
maze_t *maze;
mouse_t mouse;
for (int i = 0; i < MAX_LEVEL; ++i) {
maze = load_maze(filenames[i]);
if (maze == NULL) {
printf("Failed to load maze file %s\n", filenames[i]);
return 1;
}
printf("Level %d\n", i + 1);
mouse.x = 0;
mouse.y = 0;
play_game_with_keyboard(maze, &mouse);
mouse.x = 0;
mouse.y = 0;
play_game_with_ai(maze, &mouse);
free_maze(maze);
}
return 0;
}
```
这个程序读取三个文本文件,分别是maze1.txt、maze2.txt和maze3.txt,每个文件里保存了一个不同难度级别的迷宫地图数据。电子老鼠可以通过键盘交互方式或者AI自动走出迷宫。
阅读全文
相关推荐
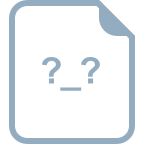


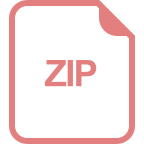
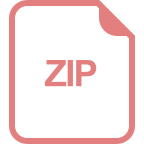
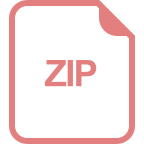
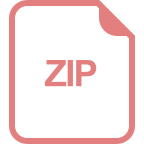
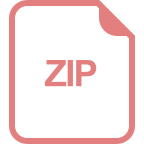
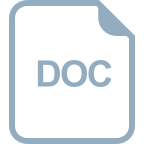
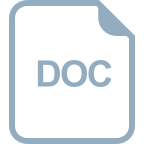
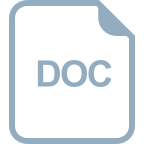
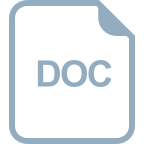
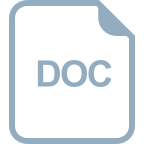
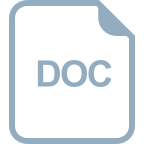



