python实现DES加解密
时间: 2023-07-07 21:18:59 浏览: 86
以下是Python中使用pycryptodome库实现DES加解密的示例代码:
```python
from Crypto.Cipher import DES
# 加密函数
def des_encrypt(key, plaintext):
cipher = DES.new(key, DES.MODE_ECB)
padded_plaintext = plaintext + (8 - len(plaintext) % 8) * chr(8 - len(plaintext) % 8)
ciphertext = cipher.encrypt(padded_plaintext.encode('utf-8'))
return ciphertext.hex()
# 解密函数
def des_decrypt(key, ciphertext):
cipher = DES.new(key, DES.MODE_ECB)
plaintext = cipher.decrypt(bytes.fromhex(ciphertext)).decode('utf-8')
plaintext = plaintext[:-ord(plaintext[-1])]
return plaintext
# 测试
key = b'abcdefgh'
plaintext = 'Hello, DES!'
ciphertext = des_encrypt(key, plaintext)
print('加密结果:', ciphertext)
decrypted_text = des_decrypt(key, ciphertext)
print('解密结果:', decrypted_text)
```
其中,`key`参数是DES算法中的密钥,长度固定为8字节;`plaintext`参数是需要加密的明文。`des_encrypt`函数返回加密后的密文,使用了ECB模式和PKCS7填充方式。`des_decrypt`函数返回解密后的明文,需要先将密文转换成字节流再进行解密。
阅读全文
相关推荐

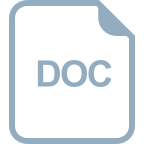
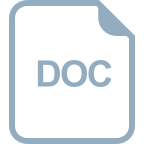
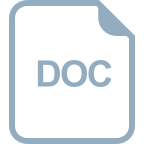




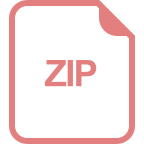