updateData.forEach(item => { if (res.code === '200') { request.put('/chongwu/update', { id: item.id, status: item.status, name: item.name, description: "123", category: "123", createtime: item.createtime, cover: item.cover, sex: item.sex, age: item.age, }).then(res => { // 处理响应结果 }); } else { this.$notify.success(res.msg) } }) } })
时间: 2023-06-11 15:06:29 浏览: 90
这段代码是一个循环,对于updateData数组中的每个元素,都会发送一个HTTP PUT请求到服务器上的/chongwu/update接口。请求中包含了一些数据,如id、status、name、description、category、createtime、cover和age等字段。如果服务器返回200状态码,那么会将响应结果处理一下;否则会通过一个消息通知组件来展示服务器返回的错误信息。
需要注意的是,这里的request变量应该是一个封装了HTTP请求的工具函数或对象,例如axios或fetch等库。另外,可以看到这里的代码逻辑比较简单,只是将数据提交到服务器上进行更新,并没有对更新结果进行进一步的处理,根据具体的业务需求,可能需要进一步的逻辑处理。
相关问题
优化这段代码 if ( this.queryParam.tonnageOne == '' || this.queryParam.tonnageTwo == '' || this.queryParam.tonnageThree == '' || this.dataSource.some((item) => !item.feiGangName) || this.dataSource.some((item) => !item.feiGangPrice) || this.dataSourceFeWater.some((item) => !item.tscfhcbName) || this.dataSourceFeWater.some((item) => !item.temp) || this.dataSourceFeWater.some((item) => !item.tscfhcb_C) || this.dataSourceFeWater.some((item) => !item.tscfhcb_Si) || this.dataSourceFeWater.some((item) => !item.tscfhcb_Mn) || this.dataSourceFeWater.some((item) => !item.tscfhcb_P) || this.dataSourceFeWater.some((item) => !item.tscfhcb_S) || this.dataSourceFeWater.some((item) => !item.tscfhcb_Price) ) { this.$message.error('请检查值,或者名称不能为空!!!') } else { this.dataSource.forEach((item) => { item['acoursewareId'] = 3343772731908188 const editableItem = this.editableData[item.id] if (editableItem) { Object.assign(item, editableItem) } }) this.editableData = [] this.dataSourceFeWater.forEach((item) => { item['acoursewareId'] = 3343772731908188 const editableItem = this.editableDataFeWater[item.id] if (editableItem) { Object.assign(item, editableItem) } }) this.editableDataFeWater = [] console.log(this.dataSourceFeWater) // 清空可编辑数据 this.conceal = false this.concealFeWater = false var addZldwInfo = { AcoursewareId: 3343772731908188, tons1: this.queryParam.tonnageOne, tons2: this.queryParam.tonnageTwo, tons3: this.queryParam.tonnageThree, } let param = { addZldwInfo: addZldwInfo, addFgList: this.dataSource, b_TscfhcbListAdd: this.dataSourceFeWater, } FeAdd(param) .then((res) => {}) .catch((error) => {}) }
你可以先将一些重复的代码提取成函数,以减少代码冗余。同时,你可以将这些验证条件提取出来,方便进行管理和修改。下面是一个简单的示例:
```javascript
function validateData(dataSource, dataSourceFeWater, queryParam) {
// 验证条件
const conditions = [
queryParam.tonnageOne === '',
queryParam.tonnageTwo === '',
queryParam.tonnageThree === '',
dataSource.some((item) => !item.feiGangName),
dataSource.some((item) => !item.feiGangPrice),
dataSourceFeWater.some((item) => !item.tscfhcbName),
dataSourceFeWater.some((item) => !item.temp),
dataSourceFeWater.some((item) => !item.tscfhcb_C),
dataSourceFeWater.some((item) => !item.tscfhcb_Si),
dataSourceFeWater.some((item) => !item.tscfhcb_Mn),
dataSourceFeWater.some((item) => !item.tscfhcb_P),
dataSourceFeWater.some((item) => !item.tscfhcb_S),
dataSourceFeWater.some((item) => !item.tscfhcb_Price),
];
if (conditions.some((condition) => condition)) {
// 验证失败
return false;
}
// 验证成功
return true;
}
function updateData(dataSource, editableData) {
dataSource.forEach((item) => {
item['acoursewareId'] = 3343772731908188;
const editableItem = editableData[item.id];
if (editableItem) {
Object.assign(item, editableItem);
}
});
editableData = [];
}
// 在原来的代码中调用新的函数
if (validateData(this.dataSource, this.dataSourceFeWater, this.queryParam)) {
updateData(this.dataSource, this.editableData);
updateData(this.dataSourceFeWater, this.editableDataFeWater);
console.log(this.dataSourceFeWater);
// 清空可编辑数据
this.conceal = false;
this.concealFeWater = false;
var addZldwInfo = {
AcoursewareId: 3343772731908188,
tons1: this.queryParam.tonnageOne,
tons2: this.queryParam.tonnageTwo,
tons3: this.queryParam.tonnageThree,
};
let param = {
addZldwInfo: addZldwInfo,
addFgList: this.dataSource,
b_TscfhcbListAdd: this.dataSourceFeWater,
};
FeAdd(param)
.then((res) => {})
.catch((error) => {});
} else {
this.$message.error('请检查值,或者名称不能为空!!!');
}
```
上面的代码,我们将验证条件和更新数据的操作分别提取成了两个函数:`validateData` 和 `updateData`。这样可以让代码更加清晰和易于维护。
优化代码 if (theData.Id.IsNullOrEmpty()) { theData.Id = Guid.NewGuid().ToSequentialGuid(); var verifyCode = theData.verifyCode; if (theData.ifNeedVerifyCode == true) { if (SessionHelper.Session["verifyCode"].IsNullOrEmpty()){ return Error("请先发送验证码"); } else if (SessionHelper.Session["verifyCode"].ToString() != verifyCode) { return Error("验证码不正确"); } } var UnWightCount= _wTapplicationBusiness.GetIQueryable(). Where(a => a.Carno == theData.Carno && a.ExpectedDate == theData.ExpectedDate && a.WeightTime.IsNullOrEmpty()) .Count(); if (UnWightCount>0) { return Error("该车在该送货日期存在未过磅的信息,请过磅后再新增数据"); } _wTapplicationBusiness.AddData(theData); _wTapplicationBusiness.GetLogger().WriteOperateLog("WTapplication信息添加", "id:" + theData.Id, true); } else { var UnWightCount = _wTapplicationBusiness.GetIQueryable(). Where(a => a.Carno == theData.Carno && a.ExpectedDate == theData.ExpectedDate && a.WeightTime.IsNullOrEmpty()&&a.Id!=theData.Id) .Count(); if (UnWightCount > 0) { return Error("该车在该送货日期存在未过磅的信息,请过磅后再新增数据"); } _wTapplicationBusiness.UpdateData(theData); _wTapplicationBusiness.GetLogger().WriteOperateLog("WTapplication信息修改", "id:" + theData.Id, true); }
可以对这段代码进行如下的优化:
```
if (theData.Id.IsNullOrEmpty())
{
theData.Id = Guid.NewGuid().ToSequentialGuid();
if (theData.ifNeedVerifyCode == true && SessionHelper.Session["verifyCode"].IsNullOrEmpty())
{
return Error("请先发送验证码");
}
if (theData.ifNeedVerifyCode == true && SessionHelper.Session["verifyCode"].ToString() != theData.verifyCode)
{
return Error("验证码不正确");
}
var unweightedCount = _wTapplicationBusiness.GetIQueryable()
.Where(a => a.Carno == theData.Carno && a.ExpectedDate == theData.ExpectedDate && a.WeightTime.IsNullOrEmpty())
.Count();
if (unweightedCount > 0)
{
return Error("该车在该送货日期存在未过磅的信息,请过磅后再新增数据");
}
_wTapplicationBusiness.AddData(theData);
_wTapplicationBusiness.GetLogger().WriteOperateLog("WTapplication信息添加", "id:" + theData.Id, true);
}
else
{
var unweightedCount = _wTapplicationBusiness.GetIQueryable()
.Where(a => a.Carno == theData.Carno && a.ExpectedDate == theData.ExpectedDate && a.WeightTime.IsNullOrEmpty() && a.Id != theData.Id)
.Count();
if (unweightedCount > 0)
{
return Error("该车在该送货日期存在未过磅的信息,请过磅后再新增数据");
}
_wTapplicationBusiness.UpdateData(theData);
_wTapplicationBusiness.GetLogger().WriteOperateLog("WTapplication信息修改", "id:" + theData.Id, true);
}
```
主要的优化点如下:
1. 合并了两个 `if` 语句,使得代码更加简洁。
2. 重命名了 `UnWightCount` 变量为 `unweightedCount`,符合命名规范。
3. 将 `_wTapplicationBusiness.GetIQueryable()` 的查询条件提取出来,避免重复写代码。
4. 将新增和修改的代码分别写在了两个分支中,使得代码更加清晰易懂。
阅读全文
相关推荐
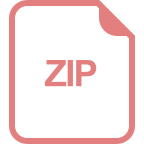
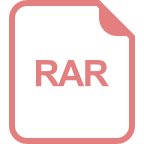
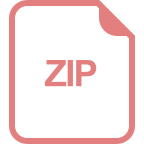













