用c语言回答以下问题,编写一个函数删除字符串中指定字符
时间: 2023-07-16 18:15:12 浏览: 100
以下是使用C语言编写的删除字符串中指定字符的函数:
```c
void delete_char(char s[], char c) {
int i, j;
for (i = 0; s[i] != '\0'; i++) {
if (s[i] == c) {
for (j = i; s[j] != '\0'; j++) {
s[j] = s[j + 1];
}
i--;
}
}
}
```
该函数接受两个参数:一个字符串s和一个字符c。它遍历字符串s中的每个字符,如果找到字符c,则将其从字符串中删除。它通过将后面的字符向前移动一个位置来实现删除。
例如,如果我们有字符串s = "hello world",并且要删除字符l,则调用函数delete_char(s, 'l')会将字符串s更改为"heo word"。
相关问题
c语言编写函数删除字符串中指定的字符
在C语言中,可以通过编写一个函数来删除字符串中指定的字符。以下是一个示例代码,展示了如何实现这个功能:
```c
#include <stdio.h>
#include <string.h>
void removeChar(char *str, char ch) {
int i, j;
int len = strlen(str);
for (i = 0; i < len; i++) {
if (str[i] == ch) {
for (j = i; j < len; j++) {
str[j] = str[j + 1];
}
len--;
i--;
}
}
}
int main() {
char str[100];
char ch;
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
str[strcspn(str, "\n")] = '\0'; // Remove newline character
printf("Enter a character to remove: ");
scanf(" %c", &ch);
removeChar(str, ch);
printf("Resulting string: %s\n", str);
return 0;
}
```
在这个示例中,`removeChar`函数接受一个字符串和一个字符作为参数,并将字符串中所有指定的字符删除。`main`函数中,我们首先从用户输入中获取一个字符串和一个要删除的字符,然后调用`removeChar`函数,最后输出结果。
C语言:编写一个函数,函数的功能是删除字符串中的所有空格
以下是一个删除字符串中所有空格的C语言函数:
```c
void remove_spaces(char* str) {
int len = strlen(str);
int i, j;
for (i = 0, j = 0; i < len; i++) {
if (str[i] != ' ') {
str[j++] = str[i];
}
}
str[j] = '\0';
}
```
这个函数使用了两个指针i和j,它们分别指向原始字符串和结果字符串中的下标。循环遍历原始字符串中的所有字符,如果当前字符不是空格,则将其复制到结果字符串中,否则跳过。最后在结果字符串的结尾添加一个空字符,以表示字符串的结束。
阅读全文
相关推荐







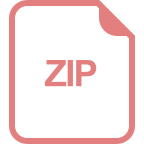







