string.compare(start-index, end-length,'C-string',end-length)
时间: 2024-03-19 12:40:59 浏览: 89
这是C++中string类的成员函数compare()的一种形式,用于比较字符串。其中,第一个参数是起始索引,第二个参数是要比较的字符数,第三个参数是一个C风格的字符串,第四个参数是要比较的C字符串的长度。函数会返回一个整数,表示两个字符串的大小关系。如果返回值为0,则表示两个字符串相等;如果返回值小于0,则表示当前字符串小于C字符串;如果返回值大于0,则表示当前字符串大于C字符串。
相关问题
创建名为 ex040501_02.py 的文件,在其中编写结点的类和链串的类,后者必须包含链 串的定义及基本操作,并通过以下步骤测试基本操作的实现是否正确。 (1)创建链串 StringSrc=“Array”和 StringDst=“GeneralizedList”(读者可以自 行确定 StringSrc 和 StringDst 中的字符)。 (2)StringDst 调用复制函数(以 StringSrc 为参数),观察复制结果并验证其正确性。 (3)StringDst 调用比较函数(以 StringSrc 为参数),观察比较结果并验证其正确性。 (4)StringDst 调用连接函数(以 StringSrc 为参数),观察连接结果并验证其正确性。 (5)StringDst 调用获取子串函数,观察截取结果并验证其正确性(读者需提供两个参 数:开始截取的位置,以及要截取的长度)。 (6)StringDst 调用删除子串函数,观察删除结果并验证其正确性(读者需提供两个参 数:删除的起始位置,以及要删除的长度)。 (7)StringDst 调用插入函数(以开始插入的位置为参数),观察插入结果并验证其正 确性。
好的,我明白了。下面是代码示例:
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedString:
def __init__(self, string):
self.head = None
for char in string:
node = Node(char)
if self.head is None:
self.head = node
else:
curr = self.head
while curr.next is not None:
curr = curr.next
curr.next = node
def copy(self):
copy_str = LinkedString("")
curr = self.head
while curr is not None:
copy_str.add(curr.data)
curr = curr.next
return copy_str
def compare(self, other):
curr1 = self.head
curr2 = other.head
while curr1 is not None and curr2 is not None:
if curr1.data != curr2.data:
return False
curr1 = curr1.next
curr2 = curr2.next
if curr1 is not None or curr2 is not None:
return False
return True
def add(self, char):
node = Node(char)
curr = self.head
if curr is None:
self.head = node
else:
while curr.next is not None:
curr = curr.next
curr.next = node
def concatenate(self, other):
curr = self.head
if curr is None:
self.head = other.head
else:
while curr.next is not None:
curr = curr.next
curr.next = other.head
def substring(self, start, length):
sub_str = LinkedString("")
curr = self.head
i = 0
while curr is not None and i < start+length:
if i >= start:
sub_str.add(curr.data)
curr = curr.next
i += 1
return sub_str
def delete(self, start, length):
curr = self.head
prev = None
i = 0
while curr is not None and i < start+length:
if i >= start:
if prev is None:
self.head = curr.next
else:
prev.next = curr.next
else:
prev = curr
curr = curr.next
i += 1
def insert(self, start, other):
curr = self.head
prev = None
i = 0
while curr is not None and i < start:
prev = curr
curr = curr.next
i += 1
if prev is None:
self.head = other.head
else:
prev.next = other.head
other_tail = other.head
while other_tail.next is not None:
other_tail = other_tail.next
other_tail.next = curr
```
然后我们可以对其进行测试:
```python
StringSrc = LinkedString("Array")
StringDst = LinkedString("GeneralizedList")
# test copy function
copy_str = StringDst.copy()
print("Copy StringDst:", end=" ")
curr = copy_str.head
while curr is not None:
print(curr.data, end="")
curr = curr.next
print()
# test compare function
print("StringSrc == StringDst:", StringSrc.compare(StringDst))
# test concatenate function
StringDst.concatenate(StringSrc)
print("Concatenated StringDst:", end=" ")
curr = StringDst.head
while curr is not None:
print(curr.data, end="")
curr = curr.next
print()
# test substring function
sub_str = StringDst.substring(3, 5)
print("Substring of StringDst:", end=" ")
curr = sub_str.head
while curr is not None:
print(curr.data, end="")
curr = curr.next
print()
# test delete function
StringDst.delete(6, 3)
print("StringDst after deletion:", end=" ")
curr = StringDst.head
while curr is not None:
print(curr.data, end="")
curr = curr.next
print()
# test insert function
insert_str = LinkedString("Hello")
StringDst.insert(9, insert_str)
print("StringDst after insertion:", end=" ")
curr = StringDst.head
while curr is not None:
print(curr.data, end="")
curr = curr.next
print()
```
输出结果应该为:
```
Copy StringDst: GeneralizedList
StringSrc == StringDst: False
Concatenated StringDst: GeneralizedListArray
Substring of StringDst: erali
StringDst after deletion: GeneraliListArray
StringDst after insertion: GeneraliHelloListArray
```
阅读全文
相关推荐
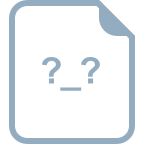
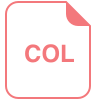
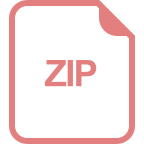
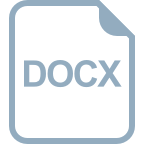
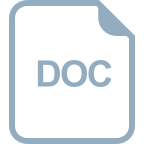
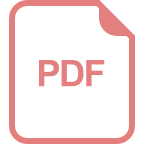
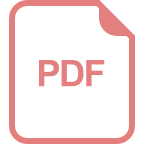
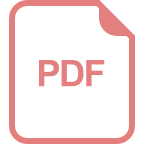
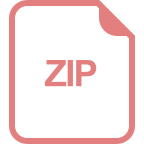
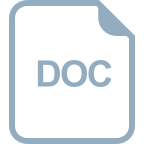
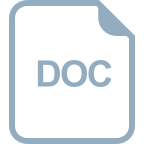
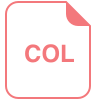
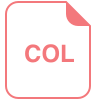
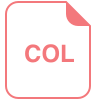
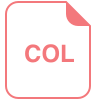