selenium查找页面表格某一列中关键字出现次数
时间: 2023-11-15 08:08:42 浏览: 85
可以使用 Selenium 先定位到表格,然后使用 BeautifulSoup 对表格进行解析,最后统计某一列中关键字出现的次数。
以下是一个示例代码,假设要统计表格中第二列中包含 "keyword" 的单元格的数量:
```python
from selenium import webdriver
from bs4 import BeautifulSoup
# 初始化浏览器
driver = webdriver.Chrome()
driver.get("https://example.com")
# 定位表格
table = driver.find_element_by_xpath("//table")
# 使用 BeautifulSoup 解析表格
soup = BeautifulSoup(table.get_attribute('outerHTML'), 'html.parser')
rows = soup.find_all('tr')
# 统计关键字出现的次数
count = 0
for row in rows:
cells = row.find_all('td')
if len(cells) > 1 and "keyword" in cells[1].get_text():
count += 1
print(count)
```
需要注意的一点是,如果表格中存在分页,需要对每一页都执行类似的代码进行统计。
相关问题
Python10中selenium4查找页面表格某一列中关键字出现次数
可以使用Selenium中的find_elements_by_xpath()方法来定位表格中的某一列,然后使用Python中的字符串函数来计算关键字出现的次数。具体实现步骤如下:
1. 先使用find_element_by_xpath()方法定位表格,然后使用find_elements_by_xpath()方法定位表格中的某一列,例如第2列可以使用如下代码:
```python
table = driver.find_element_by_xpath("//table[@id='table_id']")
col2 = table.find_elements_by_xpath(".//tr/td[2]")
```
2. 遍历列中的每一个单元格,对于每一个单元格,使用Python中的字符串函数count()来计算关键字出现的次数,例如关键字为"Python"可以使用如下代码:
```python
keyword = "Python"
count = 0
for cell in col2:
if keyword in cell.text:
count += cell.text.count(keyword)
```
完整代码如下:
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.example.com")
# 定位表格
table = driver.find_element_by_xpath("//table[@id='table_id']")
# 定位某一列
col2 = table.find_elements_by_xpath(".//tr/td[2]")
# 计算关键字出现次数
keyword = "Python"
count = 0
for cell in col2:
if keyword in cell.text:
count += cell.text.count(keyword)
print("关键字'{}'在第2列中出现了{}次".format(keyword, count))
driver.quit()
```
阅读全文
相关推荐
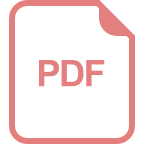
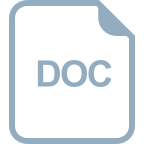
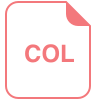
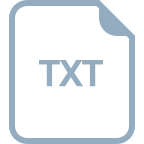
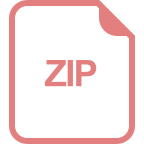
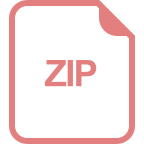
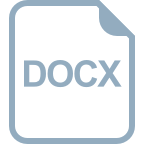
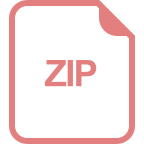
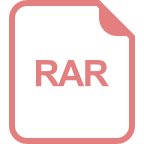
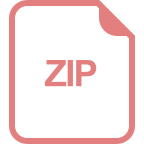
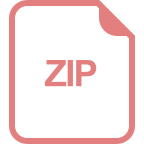
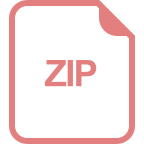
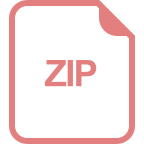
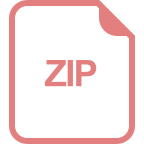