写一段自动获取时间的java代码
时间: 2023-09-18 08:06:18 浏览: 90
### 回答1:
好的,以下是获取当前时间的 Java 代码:
```java
import java.util.Date;
import java.text.SimpleDateFormat;
public class TimeGetter {
public static void main(String[] args) {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date = new Date();
String currentTime = formatter.format(date);
System.out.println("当前时间为:" + currentTime);
}
}
```
这段代码会输出当前时间,格式为"yyyy-MM-dd HH:mm:ss",例如"2022-01-01 12:00:00"。
### 回答2:
Java代码如下:
```java
import java.text.SimpleDateFormat;
import java.util.Date;
public class AutomaticTime {
public static void main(String[] args) {
// 获取当前时间
Date currentDate = new Date();
// 格式化时间
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String formattedDate = sdf.format(currentDate);
// 输出时间
System.out.println("当前时间为:" + formattedDate);
}
}
```
该代码中,我们使用`java.util.Date`类来获取当前时间,并使用`SimpleDateFormat`类来格式化时间。`SimpleDateFormat`类的构造方法需要传入一个时间格式化的模板,本例中的模板为`"yyyy-MM-dd HH:mm:ss"`,代表年份(4位)-月份-日期 小时:分钟:秒。最后,我们将格式化后的时间输出到控制台上。执行该代码,即可自动获取当前时间并显示在控制台上。
### 回答3:
下面是一段使用Java编写的自动获取时间的代码:
```
import java.text.SimpleDateFormat;
import java.util.Date;
public class AutomaticTimeGetter {
public static void main(String[] args) {
// 创建SimpleDateFormat对象,用于格式化时间
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
// 获取当前时间
Date currentTime = new Date();
// 使用SimpleDateFormat对象将时间格式化为指定格式的字符串
String formattedTime = dateFormat.format(currentTime);
// 输出格式化后的时间
System.out.println("当前时间:" + formattedTime);
}
}
```
该代码通过Java的`java.util.Date`类和`java.text.SimpleDateFormat`类实现。首先,我们创建一个`SimpleDateFormat`对象,并指定时间的格式,如`"yyyy-MM-dd HH:mm:ss"`表示年-月-日 时:分:秒。然后,使用`Date`类的`new Date()`方法获取当前时间。接着,通过`SimpleDateFormat`对象的`format()`方法将时间格式化为指定格式的字符串。最后,利用`System.out.println()`方法输出格式化后的时间。
以上代码即可实现自动获取当前时间并输出。
阅读全文
相关推荐
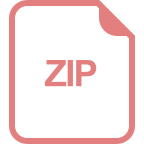
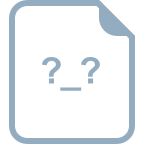
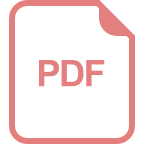
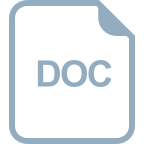
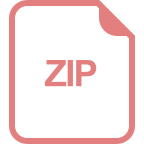
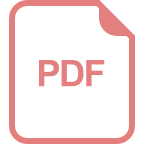
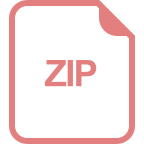
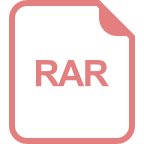
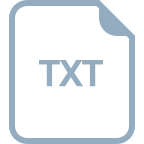
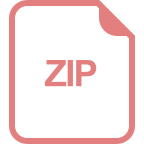
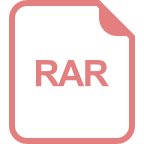
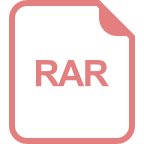
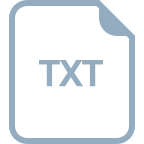
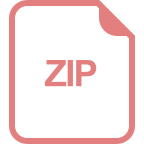
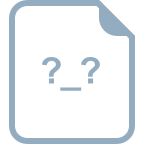