用c#语言设计一个图书管理系统,数据库的读写可以用模拟的形式实现(比如用户的账户信息可以在程序中写死,固定为账号admin,密码123456) 至少要求包含以下功能实现: 登录功能业务实现 注册功能业务实现 主菜单功能业务实现 读者类别功能业务实现 读者管理功能业务实现
时间: 2024-04-05 19:30:26 浏览: 197
好的,以下是一个简单的图书管理系统的设计,使用C#语言实现,数据库部分使用模拟的形式实现。请注意,这只是一个简单的示例,仅供参考。
1. 数据库设计
在C#中使用SQLite作为数据库。数据库中包含以下表格:
- 用户表格(user):包含用户ID、用户名、密码、用户类型等字段。
- 读者表格(reader):包含读者ID、姓名、性别、年龄、读者类别等字段。
- 图书表格(book):包含图书ID、书名、作者、出版社、ISBN码、价格、库存等字段。
- 借阅记录表格(borrow):包含借阅ID、读者ID、图书ID、借阅日期、应还日期、实际归还日期等字段。
2. 用户登录和注册模块
用户需要先进行登录,才能进行其他操作。登录时需要输入用户名和密码,系统会验证用户信息是否正确。如果用户名和密码不正确,则提示用户重新输入;如果验证通过,则跳转到主菜单页面。
如果用户还没有注册,可以点击注册按钮,进入注册页面。注册时需要填写用户名、密码、确认密码、用户类型等信息。系统会验证用户名是否已经被注册,如果没有被注册,则将用户信息保存到用户表格中,并提示用户注册成功;如果用户名已经被注册,则提示用户换一个用户名。
3. 主菜单模块
主菜单包括以下功能:
- 借阅图书:用户可以输入图书ID和读者ID来借阅图书。系统会验证图书和读者是否存在,并检查读者的借阅限制(如借阅数量、借阅时长等),如果符合要求,则将借阅记录保存到借阅记录表格中,并更新图书库存信息。
- 还书:用户可以输入借阅ID来还书。系统会验证借阅ID是否存在,并检查图书是否已经归还。如果借阅记录存在且图书未归还,则将归还日期更新到借阅记录表格中,并更新图书库存信息。
- 查询图书:用户可以输入图书ID或书名来查询图书信息。系统会从图书表格中查询相关信息,并将查询结果显示给用户。
- 查询借阅记录:用户可以输入读者ID或借阅ID来查询借阅记录。系统会从借阅记录表格中查询相关信息,并将查询结果显示给用户。
- 退出系统:用户可以退出系统,回到登录页面。
4. 读者类别模块
读者类别包括普通读者和VIP读者两种。普通读者可以借阅一定数量的图书,借阅时长也有限制;VIP读者可以借阅更多的图书,并且借阅时长更长。系统需要在读者表格中增加一个读者类别字段,并在借阅时进行相应的限制。管理员可以在读者管理模块中对读者的类别进行修改。
5. 读者管理模块
管理员可以在读者管理模块中添加、修改和删除读者信息。添加读者时需要填写读者的基本信息,并选择读者类别;修改读者时可以修改读者的基本信息和类别;删除读者时需要输入读者ID进行确认。系统会在借阅记录表格中删除与该读者相关的借阅记录,并在图书表格中更新相关图书的库存信息。
以下是C#代码示例,仅供参考:
```C#
using System;
using System.Collections.Generic;
using System.Data.SQLite;
namespace BookManagementSystem
{
class Program
{
// 数据库连接字符串
static string connString = "Data Source=book.db;Version=3;";
static void Main(string[] args)
{
Console.WriteLine("欢迎使用图书管理系统!");
while (true)
{
// 用户登录
User user = Login();
if (user == null)
{
Console.WriteLine("用户名或密码错误,请重新输入!");
continue;
}
Console.WriteLine("登录成功!");
// 进入主菜单
while (true)
{
Console.WriteLine("请选择操作:");
Console.WriteLine("1. 借阅图书");
Console.WriteLine("2. 还书");
Console.WriteLine("3. 查询图书");
Console.WriteLine("4. 查询借阅记录");
Console.WriteLine("5. 退出系统");
string choice = Console.ReadLine();
switch (choice)
{
case "1":
BorrowBook(user);
break;
case "2":
ReturnBook();
break;
case "3":
QueryBook();
break;
case "4":
QueryBorrowRecord(user);
break;
case "5":
Console.WriteLine("退出系统,谢谢使用!");
return;
default:
Console.WriteLine("无效的选项,请重新输入!");
break;
}
}
}
}
// 用户登录
static User Login()
{
Console.WriteLine("请输入用户名:");
string username = Console.ReadLine();
Console.WriteLine("请输入密码:");
string password = Console.ReadLine();
// 验证用户名和密码
using (SQLiteConnection conn = new SQLiteConnection(connString))
{
conn.Open();
string sql = "SELECT * FROM user WHERE username=@username AND password=@password";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@username", username);
cmd.Parameters.AddWithValue("@password", password);
using (SQLiteDataReader reader = cmd.ExecuteReader())
{
if (reader.Read())
{
int userId = reader.GetInt32(0);
string userType = reader.GetString(3);
return new User(userId, username, password, userType);
}
}
}
}
return null;
}
// 借阅图书
static void BorrowBook(User user)
{
Console.WriteLine("请输入图书ID:");
int bookId = int.Parse(Console.ReadLine());
Console.WriteLine("请输入读者ID:");
int readerId = int.Parse(Console.ReadLine());
// 验证图书和读者是否存在
string bookName = "";
using (SQLiteConnection conn = new SQLiteConnection(connString))
{
conn.Open();
// 验证图书
string sql = "SELECT * FROM book WHERE book_id=@book_id";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@book_id", bookId);
using (SQLiteDataReader reader = cmd.ExecuteReader())
{
if (!reader.Read())
{
Console.WriteLine("图书不存在!");
return;
}
bookName = reader.GetString(1);
int stock = reader.GetInt32(6);
if (stock <= 0)
{
Console.WriteLine("图书库存不足!");
return;
}
}
}
// 验证读者
sql = "SELECT * FROM reader WHERE reader_id=@reader_id";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@reader_id", readerId);
using (SQLiteDataReader reader = cmd.ExecuteReader())
{
if (!reader.Read())
{
Console.WriteLine("读者不存在!");
return;
}
string readerType = reader.GetString(5);
int borrowedCount = GetBorrowedCount(readerId);
if ((readerType == "普通读者" && borrowedCount >= 5) ||
(readerType == "VIP读者" && borrowedCount >= 10))
{
Console.WriteLine("借阅数量已达上限!");
return;
}
}
}
// 借阅图书
sql = "INSERT INTO borrow(reader_id, book_id, borrow_date, due_date) VALUES(@reader_id, @book_id, @borrow_date, @due_date)";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@reader_id", readerId);
cmd.Parameters.AddWithValue("@book_id", bookId);
cmd.Parameters.AddWithValue("@borrow_date", DateTime.Now.ToString("yyyy-MM-dd"));
cmd.Parameters.AddWithValue("@due_date", DateTime.Now.AddDays(30).ToString("yyyy-MM-dd"));
cmd.ExecuteNonQuery();
}
// 更新图书库存
sql = "UPDATE book SET stock=stock-1 WHERE book_id=@book_id";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@book_id", bookId);
cmd.ExecuteNonQuery();
}
Console.WriteLine("借阅成功!");
Console.WriteLine("借阅信息:");
Console.WriteLine($"读者ID:{readerId}");
Console.WriteLine($"读者姓名:{GetReaderName(readerId)}");
Console.WriteLine($"图书ID:{bookId}");
Console.WriteLine($"图书名称:{bookName}");
}
}
// 还书
static void ReturnBook()
{
Console.WriteLine("请输入借阅ID:");
int borrowId = int.Parse(Console.ReadLine());
// 验证借阅ID
int readerId = 0;
int bookId = 0;
string bookName = "";
DateTime dueDate = DateTime.Now;
using (SQLiteConnection conn = new SQLiteConnection(connString))
{
conn.Open();
// 验证借阅记录
string sql = "SELECT * FROM borrow WHERE borrow_id=@borrow_id";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@borrow_id", borrowId);
using (SQLiteDataReader reader = cmd.ExecuteReader())
{
if (!reader.Read())
{
Console.WriteLine("借阅记录不存在!");
return;
}
readerId = reader.GetInt32(1);
bookId = reader.GetInt32(2);
dueDate = reader.GetDateTime(4);
if (!reader.IsDBNull(5))
{
Console.WriteLine("图书已归还!");
return;
}
}
}
// 更新借阅记录
sql = "UPDATE borrow SET return_date=@return_date WHERE borrow_id=@borrow_id";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@borrow_id", borrowId);
cmd.Parameters.AddWithValue("@return_date", DateTime.Now.ToString("yyyy-MM-dd"));
cmd.ExecuteNonQuery();
}
// 更新图书库存
sql = "UPDATE book SET stock=stock+1 WHERE book_id=@book_id";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@book_id", bookId);
cmd.ExecuteNonQuery();
}
Console.WriteLine("还书成功!");
Console.WriteLine("借阅信息:");
Console.WriteLine($"读者ID:{readerId}");
Console.WriteLine($"读者姓名:{GetReaderName(readerId)}");
Console.WriteLine($"图书ID:{bookId}");
Console.WriteLine($"图书名称:{GetBookName(bookId)}");
Console.WriteLine($"借阅日期:{dueDate.ToString("yyyy-MM-dd")}");
Console.WriteLine($"应还日期:{dueDate.AddDays(30).ToString("yyyy-MM-dd")}");
Console.WriteLine($"归还日期:{DateTime.Now.ToString("yyyy-MM-dd")}");
}
}
// 查询图书
static void QueryBook()
{
Console.WriteLine("请输入要查询的图书ID或书名:");
string keyword = Console.ReadLine();
List<Book> books = new List<Book>();
using (SQLiteConnection conn = new SQLiteConnection(connString))
{
conn.Open();
// 查询图书
string sql = "SELECT * FROM book WHERE book_id=@book_id OR name LIKE @keyword";
using (SQLiteCommand cmd = new SQLiteCommand(sql, conn))
{
cmd.Parameters.AddWithValue("@book_id", keyword);
cmd.Parameters.AddWithValue("@keyword", $"%{keyword}%");
using (SQLiteDataReader reader = cmd.ExecuteReader())
{
while (reader.Read())
{
int bookId = reader.GetInt32(0);
string bookName = reader.GetString(1);
string author = reader.GetString(2
阅读全文
相关推荐
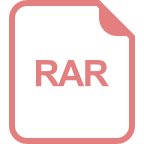
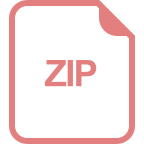
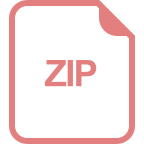
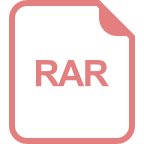
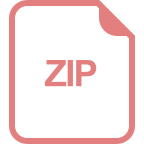
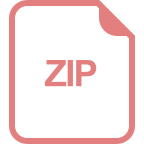
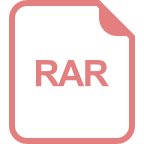
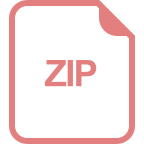
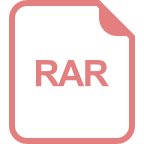
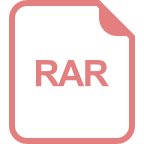
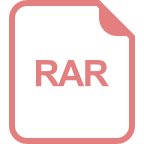
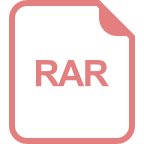
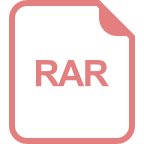
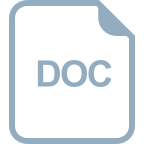