springboot+dubbo 如何使用setae
时间: 2023-12-24 20:02:37 浏览: 137
在使用SpringBoot + Dubbo框架的项目中集成Seata,需要进行以下步骤:
1. 添加Seata的相关依赖
在项目的pom.xml文件中添加Seata的相关依赖:
```xml
<dependency>
<groupId>io.seata</groupId>
<artifactId>seata-all</artifactId>
<version>${seata.version}</version>
</dependency>
```
2. 配置Seata的注册中心
在项目的application.properties或application.yml文件中添加Seata的注册中心配置,例如:
```yaml
spring:
cloud:
alibaba:
seata:
tx-service-group: my_test_tx_group
config:
type: nacos
nacos:
server-addr: localhost:8848
namespace: seata
registry:
type: nacos
nacos:
server-addr: localhost:8848
application: seata-server
```
其中,tx-service-group是Seata的事务组名称,config和registry是Seata的注册中心配置,这里使用的是Nacos作为注册中心,也可以使用其他的注册中心。
3. 配置Dubbo的Seata拦截器
在Dubbo的服务提供方和服务消费方中都需要添加Seata的拦截器,以实现分布式事务的管理。在SpringBoot + Dubbo框架中,可以通过配置文件或注解的方式来添加Seata的拦截器。
(1)配置文件方式
在Dubbo的服务提供方和服务消费方的application.properties或application.yml文件中添加Seata的拦截器配置,例如:
```yaml
# 服务提供方
dubbo.provider.filter=seata
# 服务消费方
dubbo.consumer.filter=seata
```
(2)注解方式
在Dubbo的服务提供方和服务消费方的实现类中,使用@DubboService和@DubboReference注解来定义Dubbo服务,同时在@DubboService和@DubboReference注解中添加filter属性来指定Seata的拦截器,例如:
```java
// 服务提供方
@DubboService(version = "1.0.0", filter = {"seata"})
public class OrderServiceImpl implements OrderService {
// ...
}
// 服务消费方
public class UserServiceImpl implements UserService {
@DubboReference(version = "1.0.0", filter = {"seata"})
private OrderService orderService;
// ...
}
```
4. 编写分布式事务代码
在Dubbo的服务提供方和服务消费方中,通过Seata提供的API来实现分布式事务的管理,例如:
```java
// 服务提供方
@Service
public class OrderServiceImpl implements OrderService {
@Override
@GlobalTransactional
public void createOrder(Order order) {
// 保存订单信息
orderMapper.insert(order);
// 扣减库存
storageService.reduceStock(order.getProductId(), order.getCount());
// 扣减余额
accountService.reduceBalance(order.getUserId(), order.getMoney());
}
}
// 服务消费方
@Service
public class UserServiceImpl implements UserService {
@DubboReference(version = "1.0.0")
private OrderService orderService;
@Override
@GlobalTransactional
public void createUser(User user, Order order) {
// 注册用户
userMapper.insert(user);
// 创建订单
orderService.createOrder(order);
}
}
```
在以上代码中,使用@GlobalTransactional注解来标记分布式事务的范围,Seata会根据这个注解来创建全局事务,并在所有涉及到的服务中进行事务的协调和管理。
综上所述,以上就是在SpringBoot + Dubbo框架中使用Seata进行分布式事务管理的步骤。
阅读全文
相关推荐
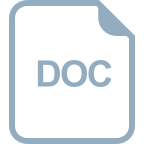
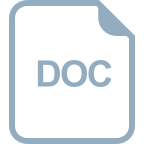
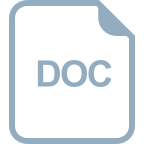
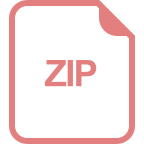
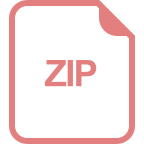
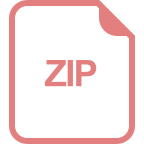
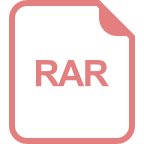
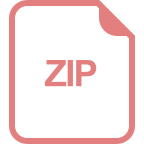
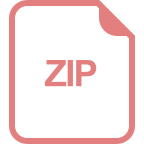
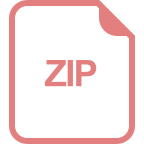
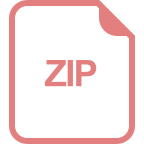
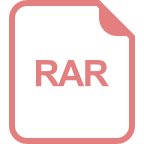
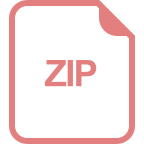
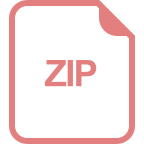
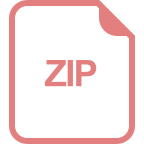
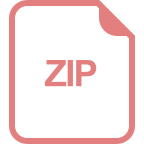


