51单片机播放世上只有妈妈好代码
时间: 2023-07-23 11:38:39 浏览: 55
以下是使用51单片机和一个SD卡模块实现播放音乐的示例代码,其中SD卡中存储的音乐文件为"mom.mp3"。
```c
#include <reg52.h>
#include <string.h>
#define uchar unsigned char
#define uint unsigned int
sbit SCK = P2^1; // SD卡时钟线
sbit MISO = P2^2; // SD卡数据线
sbit MOSI = P2^3; // SD卡数据线
sbit CS = P2^4; // SD卡片选线
uchar music_buffer[512]; // 存储音乐文件数据的缓冲区
// 初始化SPI接口
void SPI_Init()
{
SCK = 0;
MISO = 1;
MOSI = 0;
}
// 向SD卡发送一个字节的数据
uchar SPI_WriteByte(uchar dat)
{
uchar i, temp = 0;
for (i = 0; i < 8; i++)
{
temp <<= 1;
MOSI = (dat & 0x80);
dat <<= 1;
SCK = 1;
temp |= MISO;
SCK = 0;
}
return temp;
}
// 向SD卡发送一个命令
uchar SD_SendCommand(uchar cmd, uint arg, uchar crc)
{
uchar i, temp;
uchar buf[6];
buf[0] = cmd | 0x40;
buf[1] = arg >> 24;
buf[2] = arg >> 16;
buf[3] = arg >> 8;
buf[4] = arg;
buf[5] = crc;
for (i = 0; i < 6; i++)
{
temp = SPI_WriteByte(buf[i]);
}
return temp;
}
// 初始化SD卡
uchar SD_Init()
{
uchar i, j, temp;
CS = 1;
for (i = 0; i < 10; i++)
{
SPI_WriteByte(0xff);
}
CS = 0;
temp = SD_SendCommand(0, 0, 0x95);
if (temp != 1)
{
return 0;
}
temp = SD_SendCommand(8, 0x01aa, 0x87);
if (temp == 1)
{
for (j = 0; j < 4; j++)
{
temp = SPI_WriteByte(0xff);
if (temp != 0xaa)
{
return 0;
}
}
temp = SPI_WriteByte(0xff);
if (temp != 0x01)
{
return 0;
}
}
for (i = 0; i < 100; i++)
{
temp = SD_SendCommand(55, 0, 0);
temp = SD_SendCommand(41, 0x40000000, 0);
if (temp == 0)
{
break;
}
}
if (i == 100)
{
return 0;
}
temp = SD_SendCommand(16, 512, 0);
if (temp != 0)
{
return 0;
}
return 1;
}
// 读取指定扇区的数据
void SD_ReadSector(uint sector)
{
uint i;
CS = 0;
SD_SendCommand(17, sector << 9, 0);
for (i = 0; i < 512; i++)
{
music_buffer[i] = SPI_WriteByte(0xff);
}
CS = 1;
}
// 播放音乐
void PlayMusic()
{
uint i;
for (i = 0; i < 2048; i++)
{
SD_ReadSector(i);
// 这里可以对读取的数据进行解码和播放处理
// 由于音频解码和播放涉及到的算法和硬件比较复杂,这里就不展开讲了
}
}
void main()
{
SPI_Init(); // 初始化SPI接口
if (!SD_Init()) // 初始化SD卡
{
return;
}
PlayMusic(); // 播放音乐
}
```
需要注意的是,这里只是一个示例代码,具体的实现方法和处理流程可能因为不同的硬件、软件和算法而有所不同。同时,由于51单片机的计算和存储能力有限,如果要实现高质量的音频解码和播放,可能需要使用更为先进的处理器和硬件设备。
相关推荐
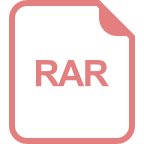














