java springboot jpa 使用缓存代码示例
时间: 2023-10-24 09:44:56 浏览: 111
当使用Spring Boot和JPA时,可以通过集成Spring Data JPA和Spring Cache来实现缓存功能。下面是一个简单的示例代码,展示了如何在Spring Boot项目中使用缓存:
首先,确保在pom.xml文件中添加必要的依赖项:
```xml
<!-- Spring Boot Starter Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- Spring Boot Starter Cache -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
接下来,在Spring Boot的配置类中启用缓存和JPA:
```java
@Configuration
@EnableCaching
@EnableJpaRepositories("com.example.repository") // 指定JPA Repository的包路径
public class AppConfig {
// 配置数据源、实体管理器等其他必要的配置
@Bean
public CacheManager cacheManager() {
return new ConcurrentMapCacheManager(); // 使用ConcurrentMap作为缓存管理器
}
}
```
然后,在需要进行缓存的方法上添加相应的注解,例如使用`@Cacheable`注解来启用缓存,并指定缓存名称:
```java
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Cacheable(value = "usersCache") // 指定缓存名称为"usersCache"
public User getUserById(Long id) {
// 从数据库中查询用户
Optional<User> userOptional = userRepository.findById(id);
return userOptional.orElse(null);
}
}
```
在上述示例中,`getUserById`方法使用了`@Cacheable`注解,并指定了缓存名称为"usersCache"。当方法被调用时,如果缓存中存在对应的结果,则直接返回缓存中的数据,否则从数据库中查询并将结果保存到缓存中。
需要注意的是,为了使缓存正常工作,确保在实体类中适当地使用`@Cacheable`注解,以标识实体是否可被缓存。
这只是一个简单的示例,你可以根据自己的需求和业务逻辑进行更复杂的缓存配置。希望对你有所帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐















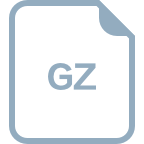
