基于andriod studio开发的失物招领页面
时间: 2024-02-03 17:02:00 浏览: 112
基于 Android Studio 开发失物招领页面需要以下步骤:
1. 创建一个 Android Studio 项目并设置基本信息。
2. 在项目中添加一个 Activity,并设置相关布局文件。
3. 添加界面元素,如文本框、按钮等。
4. 编写 Java 代码实现失物招领页面的功能,例如查询是否有匹配的失物信息、发布失物信息等。
5. 将界面与 Java 代码进行连接。
6. 测试应用程序,确保所有功能都能正常运行。
下面是一个简单的示例代码,实现了失物招领页面的基本功能:
```java
public class MainActivity extends AppCompatActivity {
private EditText editText;
private Button searchButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = (EditText) findViewById(R.id.editText);
searchButton = (Button) findViewById(R.id.searchButton);
searchButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String keyword = editText.getText().toString();
// 查询是否有匹配的失物信息
// 如果有,跳转到失物详情页面
// 如果没有,提示用户没有找到匹配的失物信息
}
});
}
}
```
在布局文件中,可以添加一个 EditText 和一个 Button,用于输入查询关键字和触发查询操作。同时,还可以添加一个 TextView 用于显示查询结果。
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:hint="输入查询关键字" />
<Button
android:id="@+id/searchButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/editText"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:text="查询" />
<TextView
android:id="@+id/resultTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/searchButton"
android:layout_marginTop="20dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:text="查询结果显示在这里" />
</RelativeLayout>
```
以上是一个简单的失物招领页面的示例代码,你可以根据自己的实际需求进行修改和完善。
阅读全文
相关推荐
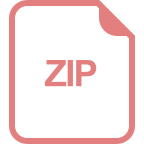
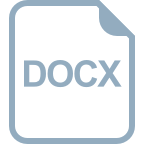
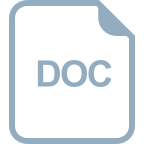

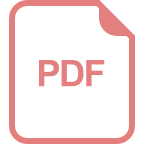
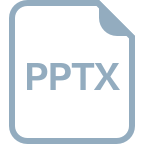
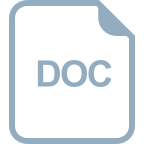
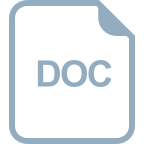
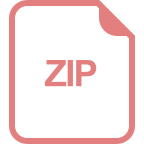
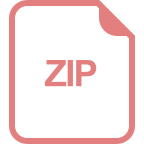
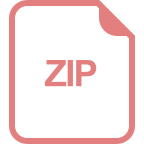
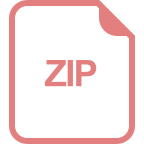


