用vue3将ruoyi封装的el-menu修改为el-cascader级联的效果
时间: 2023-11-21 09:43:32 浏览: 90
首先,我们需要安装 `element-plus`,这是 `ElementUI` 的 `Vue3` 版本。
```bash
npm install element-plus --save
```
然后,在 `main.js` 文件中引入并注册 `ElementPlus`。
```js
import { createApp } from 'vue'
import ElementPlus from 'element-plus'
import 'element-plus/lib/theme-chalk/index.css'
import App from './App.vue'
const app = createApp(App)
app.use(ElementPlus)
app.mount('#app')
```
接下来,我们需要将 `el-menu` 组件封装成一个新的组件 `el-cascader-menu`,并在其中使用 `el-cascader` 组件来实现级联选择效果。
```html
<template>
<el-cascader
v-model="selected"
:options="options"
:props="props"
:show-all-levels="false"
@change="handleChange"
></el-cascader>
</template>
<script>
import { ref, watch } from 'vue'
import { useRoute } from 'vue-router'
export default {
name: 'ElCascaderMenu',
props: {
menuList: {
type: Array,
required: true
}
},
setup(props) {
const route = useRoute()
const selected = ref([])
const options = ref(props.menuList)
const propsData = {
checkStrictly: true,
value: 'id',
label: 'title',
children: 'children'
}
const props = ref(propsData)
watch(
() => props.value,
() => {
options.value = props.menuList
}
)
const handleChange = (value) => {
const path = value.map((id) => {
const item = options.value.find((item) => item.id === id)
return item.path || item.url
})
route.push(path.join('/'))
}
return {
selected,
options,
props,
handleChange
}
}
}
</script>
```
在 `setup` 函数中,我们使用 `ref` 创建了 `selected`、`options`、`props` 三个响应式变量,并使用 `watch` 监听 `props.value` 的变化来更新 `options` 的值。
`handleChange` 函数用来处理选择项的变化,并使用 `vue-router` 来实现路由跳转。
最后,我们需要在 `App.vue` 文件中引入并使用 `el-cascader-menu` 组件。
```html
<template>
<div id="app">
<el-cascader-menu :menuList="menuList" />
</div>
</template>
<script>
import ElCascaderMenu from '@/components/ElCascaderMenu.vue'
export default {
name: 'App',
components: {
ElCascaderMenu
},
data() {
return {
menuList: [
{
id: '1',
title: '导航1',
children: [
{
id: '1-1',
title: '选项1',
children: [
{
id: '1-1-1',
title: '选项1-1',
url: '/option-1-1'
},
{
id: '1-1-2',
title: '选项1-2',
url: '/option-1-2'
}
]
},
{
id: '1-2',
title: '选项2',
url: '/option-2'
}
]
},
{
id: '2',
title: '导航2',
children: [
{
id: '2-1',
title: '选项1',
url: '/option-2-1'
},
{
id: '2-2',
title: '选项2',
url: '/option-2-2'
}
]
}
]
}
}
}
</script>
```
在 `menuList` 中,我们使用了 `children` 字段来实现级联选择的效果。当某一层级的选项被选中后,其子选项会自动展示在下一层级中。
最终效果如下所示:

相关推荐
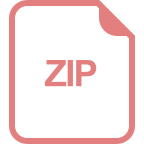
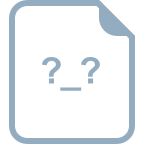














