c++中#include<stdlib.h>用来干嘛
时间: 2023-11-22 11:05:19 浏览: 34
c是一种编程语言,它是由贝尔实验室的Dennis Ritchie在1972年开发的。c是一种结构化的、高效的、通用的编程语言,被广泛用于系统软件、应用软件、嵌入式系统和游戏开发等领域。c语言具有强大的指针和内存管理能力,可以直接操作硬件和系统资源。许多其他编程语言,如C++、Java和Python等,都受到了c语言的影响。
相关问题
include <stdio.h>#include <stdlib.h>
这是一个C语言的标准头文件的引用,其中<stdio.h>包含了C语言的输入输出函数,而<stdlib.h>包含了一些通用的工具函数和内存分配函数。这些头文件在C语言中非常常见,几乎每个C程序都会用到它们。下面是一个简单的例子,展示了如何使用这两个头文件。
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
char str[100];
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered %d.\n", num);
printf("Enter a string: ");
scanf("%s", str);
printf("You entered %s.\n", str);
return 0;
}
```
上面的程序使用了<stdio.h>中的printf和scanf函数,以及<stdlib.h>中的exit函数。输入一个整数和一个字符串,然后将它们输出到控制台上。
#include<stdio.h> #include<stdlib.h>
#include<stdio.h>和#include<stdlib.h>是C语言中的预处理指令,用于引入标准输入输出函数和动态内存分配函数。它们通常一起使用,但有时候会出现报错的情况。
报错的原因可能是由于编译器或IDE的设置问题,或者是代码中的其他错误导致的。在某些情况下,删除#include<stdlib.h>可能会解决报错问题,但这并不是一个通用的解决方案。
为了解决这个问题,你可以尝试以下几个步骤:
1. 确保你的编译器或IDE已正确配置,并且能够找到正确的头文件路径。
2. 检查你的代码中是否存在其他错误,例如语法错误、函数声明错误等。
3. 尝试使用不同的编译器或IDE来编译你的代码,看是否能够解决问题。
4. 如果以上步骤都没有解决问题,你可以尝试在代码中手动声明所需的函数,而不使用#include<stdlib.h>。例如,你可以在代码中添加以下声明:
```c
void *malloc(size_t size);
void free(void *ptr);
```
请注意,这只是一种临时解决方案,如果你的代码依赖于stdlib.h中的其他函数或定义,可能会导致其他问题。
相关推荐
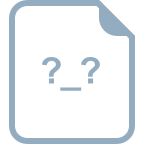
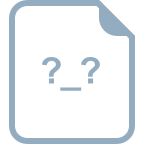
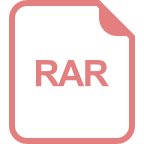












