用C语言写一个带注释的双向链表
时间: 2023-09-23 15:12:10 浏览: 36
好的,下面是一个带注释的双向链表的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* prev;
struct Node* next;
};
struct Node* head = NULL;
void insert(int value) {
// 创建新节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->prev = NULL;
newNode->next = NULL;
if (head == NULL) {
// 如果链表为空,将新节点作为头节点
head = newNode;
} else {
// 找到最后一个节点
struct Node* lastNode = head;
while (lastNode->next != NULL) {
lastNode = lastNode->next;
}
// 插入新节点
lastNode->next = newNode;
newNode->prev = lastNode;
}
}
void delete(int value) {
// 找到要删除的节点
struct Node* currNode = head;
while (currNode != NULL && currNode->data != value) {
currNode = currNode->next;
}
if (currNode == NULL) {
// 要删除的节点不存在
printf("Error: The node to be deleted does not exist.\n");
return;
}
if (currNode == head) {
// 如果要删除的是头节点
head = currNode->next;
if (head != NULL) {
head->prev = NULL;
}
} else {
// 如果要删除的是中间或尾部节点
currNode->prev->next = currNode->next;
if (currNode->next != NULL) {
currNode->next->prev = currNode->prev;
}
}
// 释放要删除的节点的内存
free(currNode);
}
void printList() {
// 遍历链表并打印每个节点的值
struct Node* currNode = head;
while (currNode != NULL) {
printf("%d ", currNode->data);
currNode = currNode->next;
}
printf("\n");
}
int main() {
// 插入一些节点
insert(1);
insert(2);
insert(3);
insert(4);
insert(5);
// 打印链表
printf("Original List: ");
printList();
// 删除节点
delete(3);
delete(5);
// 打印修改后的链表
printf("Modified List: ");
printList();
// 退出程序前,释放所有节点的内存
struct Node* currNode = head;
while (currNode != NULL) {
struct Node* nextNode = currNode->next;
free(currNode);
currNode = nextNode;
}
return 0;
}
```
注释中对每段代码的功能进行了解释,包括新节点的插入、节点的删除、链表的遍历、以及在程序退出前释放所有节点的内存。
相关推荐
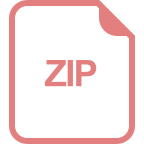
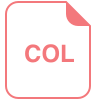
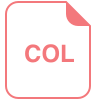
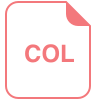
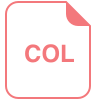
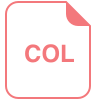









