binance写一个macd策略
时间: 2023-06-12 07:05:02 浏览: 62
MACD(Moving Average Convergence Divergence)是一种常用的技术指标,用于分析股票价格的趋势和动量。MACD指标包括两条线:MACD线和信号线。当MACD线跨越信号线时,这通常被视为一个买入或卖出信号。
以下是一个使用Binance API和TA-Lib库编写的MACD策略示例,该示例基于Python编写,并使用Binance的历史价格数据进行分析:
```python
import numpy as np
import talib
import pandas as pd
import ccxt
# Initialize the Binance exchange
binance = ccxt.binance()
# Define the symbol and timeframe
symbol = 'BTC/USDT'
timeframe = '1d'
# Define the number of periods for the MACD calculation
fast_period = 12
slow_period = 26
signal_period = 9
# Download historical data from Binance
ohlcv = binance.fetch_ohlcv(symbol, timeframe)
df = pd.DataFrame(ohlcv, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume'])
df['timestamp'] = pd.to_datetime(df['timestamp'], unit='ms')
df.set_index('timestamp', inplace=True)
# Calculate the MACD and signal line using TA-Lib
macd, signal, hist = talib.MACD(df['close'], fastperiod=fast_period, slowperiod=slow_period, signalperiod=signal_period)
# Determine the buy/sell signals
df['macd'] = macd
df['signal'] = signal
df['hist'] = hist
df['macd_cross'] = np.where(df['macd'] > df['signal'], 1, 0)
df['macd_cross'] = np.where(df['macd'] < df['signal'], -1, df['macd_cross'])
# Print the dataframe with MACD and signal line
print(df[['close', 'macd', 'signal', 'hist', 'macd_cross']])
# Plot the MACD and signal line
import matplotlib.pyplot as plt
plt.plot(df['macd'], label='MACD')
plt.plot(df['signal'], label='Signal Line')
plt.legend()
plt.show()
```
在上面的示例中,我们首先初始化了Binance交易所,并指定了要分析的交易对和时间帧。然后,我们使用Binance API从交易所下载历史价格数据,并使用TA-Lib库计算MACD和信号线。接下来,我们确定了买入和卖出信号,并将其添加到数据帧中。最后,我们打印了数据帧,并使用matplotlib库绘制了MACD和信号线的图表。
请注意,这只是一个简单的示例,MACD策略可能需要更复杂的过滤器和风险管理技巧。在使用任何交易策略之前,请务必进行充分的测试和风险评估。
相关推荐
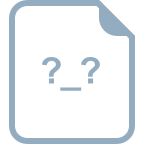














