用Pthreads实现梯形积分法
时间: 2024-02-03 11:11:42 浏览: 86
梯形积分法是一种数值积分方法,可以用来近似计算定积分。在使用Pthreads实现梯形积分法时,可以将计算任务分成多个子任务,每个子任务使用梯形积分法来计算一部分定积分,然后将所有子任务的计算结果汇总得到最终的积分值。
下面是一个使用Pthreads实现梯形积分法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <math.h>
#define NUM_THREADS 4 // 线程数量
#define N 10000000 // 划分区间数
#define a 0.0 // 积分下限
#define b 1.0 // 积分上限
double f(double x) {
return sin(x); // 被积函数
}
double trap(double left, double right, double height) {
return (left + right) * height / 2.0; // 梯形面积公式
}
void *worker(void *arg) {
int tid = *(int*)arg;
double h = (b - a) / N; // 子区间宽度
int start = tid * N / NUM_THREADS; // 子区间起始索引
int end = (tid + 1) * N / NUM_THREADS; // 子区间结束索引
double sum = 0.0;
for (int i = start; i < end; i++) {
double left = a + i * h;
double right = a + (i + 1) * h;
double height = (f(left) + f(right)) / 2.0;
sum += trap(f(left), f(right), h);
}
double *result = malloc(sizeof(double));
*result = sum;
return (void*)result;
}
int main() {
pthread_t threads[NUM_THREADS];
int tids[NUM_THREADS];
double sum = 0.0;
for (int i = 0; i < NUM_THREADS; i++) {
tids[i] = i;
pthread_create(&threads[i], NULL, worker, (void*)&tids[i]);
}
for (int i = 0; i < NUM_THREADS; i++) {
double *result;
pthread_join(threads[i], (void**)&result);
sum += *result;
free(result);
}
printf("Integral value: %f\n", sum);
return 0;
}
```
在这个示例中,我们定义了一个`worker`函数来执行每个子任务的计算。该函数需要接受一个整数参数,表示线程的ID。在该函数中,我们首先计算出子区间的起始索引和结束索引,然后通过梯形积分法来计算该子区间的积分值。最后,将计算结果存储在堆上分配的内存中,并将其作为返回值返回。
在`main`函数中,我们首先创建了多个线程,并分别为每个线程传递一个唯一的线程ID。然后,我们使用`pthread_join`函数来等待每个线程的计算结果,并将这些结果相加得到最终的积分值。最后,我们输出积分值并退出程序。
需要注意的是,在使用Pthreads实现梯形积分法时,需要将任务平均分配给每个线程,并确保每个线程都处理不同的区间。此外,由于每个线程都会分配堆上的内存,因此在使用完毕后需要及时释放这些内存,以避免内存泄漏问题。
阅读全文
相关推荐






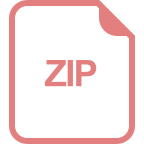
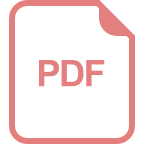
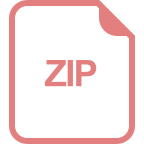
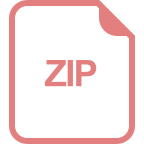
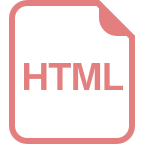
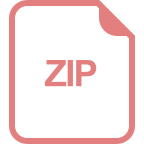
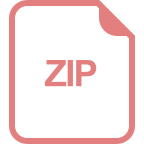