asp.net,js 如何调用C#中的函数
时间: 2024-03-09 09:48:51 浏览: 21
在 ASP.NET 中,可以使用 Code-behind 文件来编写 C# 代码,并在前端页面中调用这些代码中的函数。具体步骤如下:
1. 在 ASP.NET 项目中创建一个 Code-behind 文件,比如 Default.aspx.cs。
2. 在 Code-behind 文件中定义一个公共函数,比如:
```
public void MyFunction(string param)
{
// Do something with param
}
```
3. 在前端页面中添加一个按钮或者其他触发事件,比如:
```
<asp:Button ID="btnSubmit" runat="server" OnClick="btnSubmit_Click" Text="Submit" />
```
4. 在前端页面对应的 Code-behind 文件中,添加一个事件处理函数,并在其中调用之前定义的公共函数,比如:
```
protected void btnSubmit_Click(object sender, EventArgs e)
{
string myParam = "Hello";
MyFunction(myParam);
}
```
在 JavaScript 中调用 C# 函数则需要使用 Ajax 技术。具体步骤如下:
1. 在 C# 中创建一个 WebMethod,使用 [WebMethod] 标志进行标记,比如:
```
[WebMethod]
public static string MyFunction(string param)
{
// Do something with param
return "Result";
}
```
2. 在 JavaScript 中使用 Ajax 发送一个 POST 请求到 C# 的 WebMethod,比如:
```
$.ajax({
type: "POST",
url: "MyPage.aspx/MyFunction",
data: JSON.stringify({ param: "Hello" }),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (result) {
alert(result.d);
}
});
```
其中,MyPage.aspx 是包含 MyFunction 的 ASP.NET 页面的名称,result.d 是返回的结果。
相关推荐
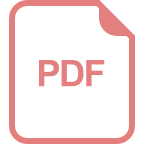
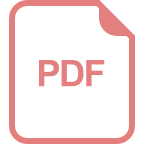
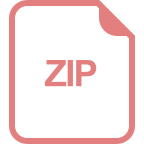














